输入一个字符串,判断其是否为回文串,用C语言
时间: 2024-12-06 20:23:36 浏览: 12
在C语言中,判断一个字符串是否为回文串可以采用双指针法,即从字符串的两端开始比较字符,直到两个指针相遇或交叉。如果在整个过程中所有字符都相等,则该字符串是回文串;否则不是。这里是一个简单的C语言函数示例:
```c
#include <stdbool.h>
#include <string.h>
bool isPalindrome(char str[]) {
int left = 0;
int right = strlen(str) - 1; // 获取字符串长度减一作为右边界
while (left < right) { // 只要左指针小于右指针,就继续比较
if (str[left] != str[right]) { // 如果左右字符不等,返回false
return false;
}
left++; // 左指针向右移动
right--; // 右指针向左移动
}
return true; // 比较结束后,字符串是回文,返回true
}
// 测试函数
int main() {
char test_str[] = "madam"; // 测试字符串
if (isPalindrome(test_str)) {
printf("%s is a palindrome.\n", test_str);
} else {
printf("%s is not a palindrome.\n", test_str);
}
return 0;
}
```
在这个例子中,`isPalindrome` 函数接收一个字符串 `str` 的地址,并通过两个指针 `left` 和 `right` 对比字符,判断是否为回文。
阅读全文
相关推荐
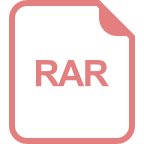
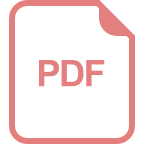















