python花卉图像识别系统具体代码
时间: 2024-12-26 08:11:10 浏览: 0
Python花卉图像识别系统的代码可能会涉及到很多方面,包括图像处理、深度学习模型训练和部署等。一般而言,这个系统的实现可以分为以下几个步骤:
1. 数据准备:获取花卉图像数据集,并对其进行预处理,如图像增强、数据清洗等。
2. 特征提取:使用图像处理技术提取花卉图像的特征向量,如使用卷积神经网络(CNN)提取图像特征。
3. 模型训练:使用深度学习框架如TensorFlow、Keras或PyTorch等进行模型训练,并对模型进行优化和调参。
4. 模型部署:将训练好的模型部署到web应用或移动端应用中,以进行花卉识别。
以下是一些参考代码:
数据准备:
```
import os
import cv2
import numpy as np
def load_data(data_dir):
images = []
labels = []
for label, name in enumerate(os.listdir(data_dir)):
for filename in os.listdir(os.path.join(data_dir, name)):
img_path = os.path.join(data_dir, name, filename)
img = cv2.imread(img_path)
img = cv2.resize(img, (224, 224))
images.append(img)
labels.append(label)
return np.array(images), np.array(labels)
```
特征提取:
```
from tensorflow.keras.applications.resnet50 import preprocess_input
from tensorflow.keras.applications import ResNet50
def extract_features(images):
model = ResNet50(weights='imagenet', include_top=False, pooling='avg')
images = preprocess_input(images)
features = model.predict(images)
return features
```
模型训练:
```
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout
def train_model(train_features, train_labels, num_classes):
model = Sequential()
model.add(Dense(256, activation='relu', input_dim=train_features.shape))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
model.fit(train_features, train_labels, epochs=10, batch_size=32)
return model
```
模型部署:
```
from tensorflow.keras.models import load_model
def predict_flower(image_path):
image = cv2.imread(image_path)
image = cv2.resize(image, (224, 224))
image = preprocess_input(np.expand_dims(image, axis=0))
model = load_model('flower_model.h5')
predictions = model.predict(image)
predicted_class = np.argmax(predictions)
return predicted_class
```
阅读全文
相关推荐
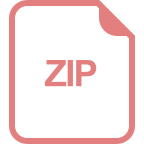
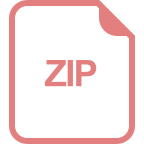
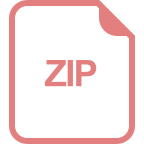
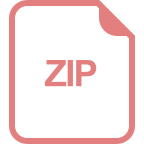
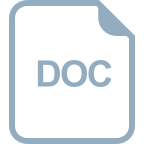
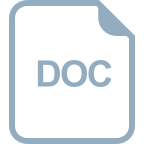
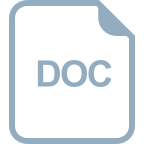
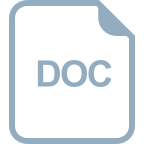







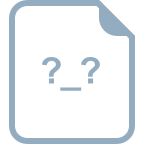
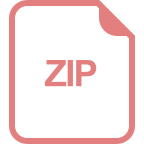
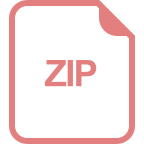
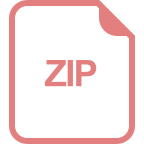