How to make tmdq progress bar for multilprocess
时间: 2024-10-25 18:07:27 浏览: 14
To create a TMDQ (Terminal Multiline Display Queue) progress bar for multiprocessing in Python, you can use the `tqdm` library, which provides an easy-to-use interface for displaying progress bars and is compatible with multiprocessing tasks. Here's a simple example on how to do it:
1. **Install tqdm**: If you haven't installed `tqdm`, you can do so using pip:
```
pip install tqdm
```
2. **Import necessary libraries**:
```python
from multiprocessing import Process
from tqdm import tqdm
```
3. **Create a function that performs some process and updates the progress bar**:
```python
def worker(counter, total):
for i in tqdm(range(total), desc="Processing", unit='tasks'):
# Your processing code here
time.sleep(0.1) # Simulate some work
counter.value += 1
```
In this example, we use `desc` for the description of the progress bar and `unit` for the unit of progression.
4. **Use `multiprocessing.Process` and `Manager()` objects**:
```python
manager = Manager()
counter = manager.Value('i', 0)
processes = []
# Create multiple processes
for _ in range(num_processes):
p = Process(target=worker, args=(counter, total_tasks))
processes.append(p)
p.start()
# Wait for all processes to finish
for p in processes:
p.join()
print("All tasks completed.")
```
5. **The progress bar will update automatically as each process calls the `counter.value += 1` line**. Make sure each process has access to the shared `counter` object through the `manager`.
Here are a few related questions:
阅读全文
相关推荐
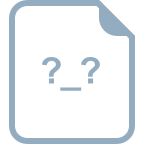
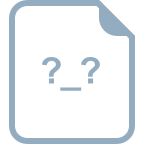
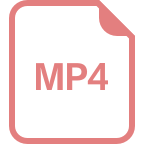
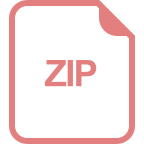
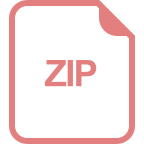
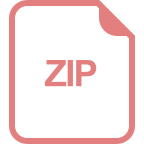