用python编写LSTM代码实现负荷预测
时间: 2023-09-16 08:12:15 浏览: 113
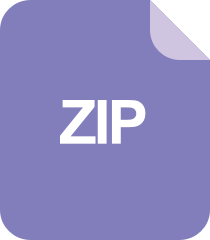
python实现的基于LSTM的电力负荷预测源码+全部数据.zip

好的,以下是用Python编写LSTM代码实现负荷预测的基本步骤:
Step 1: 导入所需的库
```
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.preprocessing import MinMaxScaler
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, LSTM
```
Step 2: 读取并预处理数据
读取负荷预测数据并对数据进行标准化处理:
```
# 读取负荷预测数据
data = pd.read_csv("[你的数据文件路径]")
# 取出负荷数据,并将其转换成numpy数组
raw_data = data.iloc[:, 1].values.reshape(-1, 1)
# 将负荷数据标准化
scaler = MinMaxScaler()
data = scaler.fit_transform(raw_data)
```
Step 3: 划分训练集和测试集
```
# 把数据划分为训练集和测试集
train_size = int(len(data) * 0.8)
test_size = len(data) - train_size
train, test = data[0:train_size,:], data[train_size:len(data),:]
```
Step 4: 创建LSTM模型
```
# 创建一个LSTM模型
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(train.shape[1], 1)))
model.add(LSTM(units=50))
model.add(Dense(1))
```
Step 5: 编译模型
```
# 编译模型
model.compile(loss='mean_squared_error', optimizer='adam')
```
Step 6: 拟合模型并预测数据
```
# 拟合模型
history = model.fit(trainX, trainY, epochs=100, batch_size=64, validation_data=(testX, testY), verbose=2, shuffle=False)
# 预测测试集负荷
trainPredict = model.predict(trainX)
testPredict = model.predict(testX)
# 反标准化预测数据
trainPredict = scaler.inverse_transform(trainPredict)
testPredict = scaler.inverse_transform(testPredict)
```
以上就是用Python编写LSTM代码实现负荷预测的基本步骤,希望能对你有所帮助。
阅读全文
相关推荐
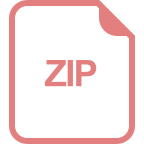
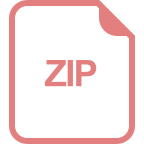


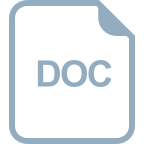
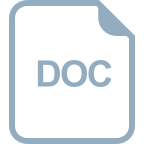
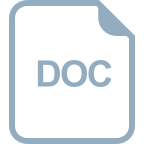
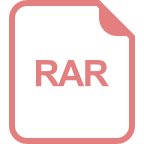
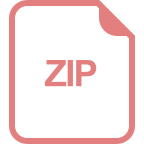
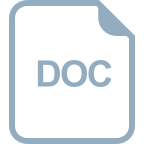
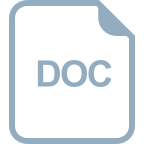
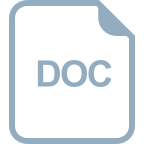
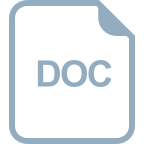
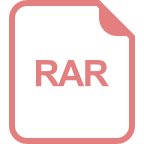