javaBea+jsp实现复数的4则运算
时间: 2024-02-23 12:59:42 浏览: 24
以下是JavaBean和JSP实现复数四则运算的示例代码:
Complex.java (JavaBean)
```java
public class Complex {
private double real;
private double imag;
public Complex() {
this.real = 0.0;
this.imag = 0.0;
}
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImag() {
return imag;
}
public void setImag(double imag) {
this.imag = imag;
}
public Complex add(Complex other) {
double real = this.real + other.real;
double imag = this.imag + other.imag;
return new Complex(real, imag);
}
public Complex subtract(Complex other) {
double real = this.real - other.real;
double imag = this.imag - other.imag;
return new Complex(real, imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
@Override
public String toString() {
return String.format("%.2f + %.2fi", real, imag);
}
}
```
complex.jsp
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="com.example.Complex" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Complex Calculator</title>
</head>
<body>
<h1>Complex Calculator</h1>
<%-- 获取表单参数 --%>
<%
double real1 = Double.parseDouble(request.getParameter("real1"));
double imag1 = Double.parseDouble(request.getParameter("imag1"));
double real2 = Double.parseDouble(request.getParameter("real2"));
double imag2 = Double.parseDouble(request.getParameter("imag2"));
Complex c1 = new Complex(real1, imag1);
Complex c2 = new Complex(real2, imag2);
Complex result = null;
String operator = request.getParameter("operator");
if (operator.equals("+")) {
result = c1.add(c2);
} else if (operator.equals("-")) {
result = c1.subtract(c2);
} else if (operator.equals("*")) {
result = c1.multiply(c2);
} else if (operator.equals("/")) {
result = c1.divide(c2);
}
%>
<form method="post">
<fieldset>
<legend>Complex Number 1</legend>
<label>Real:</label>
<input type="text" name="real1" value="<%=real1%>"/><br/>
<label>Imaginary:</label>
<input type="text" name="imag1" value="<%=imag1%>"/><br/>
</fieldset>
<fieldset>
<legend>Complex Number 2</legend>
<label>Real:</label>
<input type="text" name="real2" value="<%=real2%>"/><br/>
<label>Imaginary:</label>
<input type="text" name="imag2" value="<%=imag2%>"/><br/>
</fieldset>
<fieldset>
<legend>Operator</legend>
<input type="radio" name="operator" value="+" <%=operator.equals("+") ? "checked" : ""%>> +<br>
<input type="radio" name="operator" value="-" <%=operator.equals("-") ? "checked" : ""%>> -<br>
<input type="radio" name="operator" value="*" <%=operator.equals("*") ? "checked" : ""%>> *<br>
<input type="radio" name="operator" value="/" <%=operator.equals("/") ? "checked" : ""%>> /<br>
</fieldset>
<input type="submit" value="Calculate"/>
</form>
<%
if (result != null) {
out.println("<h2>Result: " + result + "</h2>");
}
%>
</body>
</html>
```
在此示例中,我们使用JavaBean(Complex类)来表示复数,并在JSP中实现四则运算。JSP页面包括一个表单,用户可以输入两个复数和运算符。当用户提交表单时,JSP页面会将表单参数传递给JavaBean,并调用相应的方法计算结果。最后,JSP页面会显示结果。
需要注意的是,此示例仅用于演示目的。在实际应用中,需要对用户输入进行验证和错误处理,以确保应用程序的正确性和安全性。
相关推荐
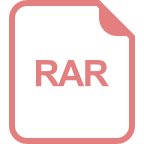
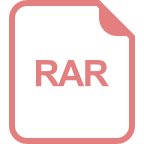




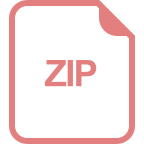
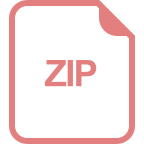
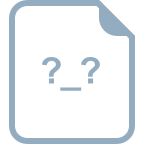
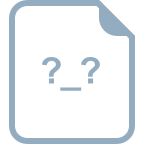