Python 多态练习题
时间: 2023-10-26 08:17:56 浏览: 62
好的,以下是几个 Python 多态的练习题:
1. 创建一个 `Animal` 类和它的子类 `Dog`、`Cat`。每个子类都有一个 `speak()` 方法,分别返回 `"Woof"` 和 `"Meow"`。创建一个函数 `animal_speak()`,它接受一个 `Animal` 类型的对象,并调用它的 `speak()` 方法。
```python
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof"
class Cat(Animal):
def speak(self):
return "Meow"
def animal_speak(animal):
return animal.speak()
```
2. 创建一个 `Shape` 类和它的子类 `Circle`、`Rectangle`。每个子类都有一个 `area()` 方法,分别返回圆形和矩形的面积。创建一个函数 `get_area()`,它接受一个 `Shape` 类型的对象,并调用它的 `area()` 方法。
```python
import math
class Shape:
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def get_area(shape):
return shape.area()
```
3. 创建一个 `Person` 类和它的子类 `Student`、`Teacher`。每个子类都有一个 `teach()` 方法,分别返回 `"I will teach you"` 和 `"I will grade your papers"`. 创建一个函数 `classroom()`,它接受一个 `Person` 类型的对象,并调用它的 `teach()` 方法。
```python
class Person:
def teach(self):
pass
class Student(Person):
def teach(self):
return "I will learn from you"
class Teacher(Person):
def teach(self):
return "I will teach you"
def classroom(person):
return person.teach()
```
这些练习题可以帮助你加深对 Python 多态的理解。
相关推荐
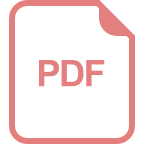
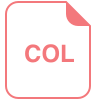
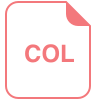
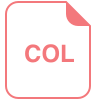
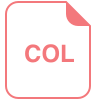










