csharp 生成pdf代码示例
时间: 2023-09-25 15:09:42 浏览: 67
很高兴为您解答问题。以下是一个简单的C#生成pdf文件的示例代码:
using System;
using System.IO;
using iTextSharp.text;
using iTextSharp.text.pdf;
namespace GeneratePDF
{
class Program
{
static void Main(string[] args)
{
Document doc = new Document();
PdfWriter.GetInstance(doc, new FileStream("output.pdf", FileMode.Create));
doc.Open();
Paragraph paragraph = new Paragraph("Hello World!");
doc.Add(paragraph);
doc.Close();
Console.WriteLine("PDF文件生成成功!");
}
}
}
以上代码使用iTextSharp库生成一个最简单的PDF文件。您可以根据自己的需要进行修改和扩展。希望能帮助到您。
相关问题
.net使用开源组件把word转为图片或者pdf代码示例
### 回答1:
你可以使用 Spire.Doc for .NET 库来将 Word 文档转换为图片或 PDF。下面是一个示例代码,它可以将 Word 文档转换为 PDF:
```
using Spire.Doc;
using Spire.Doc.Documents;
namespace WordToPdf
{
class Program
{
static void Main(string[] args)
{
// Load the Word document
Document document = new Document();
document.LoadFromFile("input.docx");
// Save the document as a PDF file
document.SaveToFile("output.pdf", FileFormat.PDF);
}
}
}
```
要将 Word 文档转换为图片,可以使用 Spire.Doc for .NET 中的 `ToImage()` 方法。下面是一个示例代码,它可以将 Word 文档的第一页转换为 JPEG 格式的图片:
```
using Spire.Doc;
using Spire.Doc.Documents;
using Spire.Doc.Fields;
namespace WordToImage
{
class Program
{
static void Main(string[] args)
{
// Load the Word document
Document document = new Document();
document.LoadFromFile("input.docx");
// Get the first page of the document
Page page = document.Pages[0];
// Convert the page to an image and save it
page.ToImage().Save("output.jpg", ImageFormat.Jpeg);
}
}
}
```
希望这些代码能帮到你!
### 回答2:
使用开源组件将Word转换为图片或PDF可以借助于Aspose组件库。Aspose.Words for .NET是一款功能强大的API,可以通过以下示例代码来实现Word到图片或PDF的转换:
首先,确保已在项目中引入Aspose.Words for .NET组件。然后,可以按照以下步骤进行转换:
1. 将Word文档加载到Aspose.Words文档对象中:
```csharp
Document doc = new Document("input.docx");
```
2. 将Word文档保存为图片:
```csharp
for (int i = 0; i < doc.PageCount; i++)
{
using (MemoryStream stream = new MemoryStream())
{
ImageSaveOptions options = new ImageSaveOptions(SaveFormat.Jpeg);
options.PageIndex = i;
doc.Save(stream, options);
// 保存图片到本地
using (FileStream fileStream = new FileStream($"output{i}.jpg", FileMode.Create))
{
stream.Position = 0;
stream.CopyTo(fileStream);
}
}
}
```
3. 将Word文档保存为PDF:
```csharp
doc.Save("output.pdf", SaveFormat.Pdf);
```
以上示例代码将Word文档逐页转换为图片或直接转换为PDF,并保存到本地。你可以根据需求进行调整,如设置输出图片的格式、质量、页面范围等。
同时,由于Aspose.Words是一个商业软件,使用时需要购买许可证或使用试用版。
### 回答3:
在使用.NET将Word转为图片或PDF时,可以利用开源组件来实现。下面是一个使用开源组件Aspose.Words的示例代码:
```csharp
using Aspose.Words;
using System.Drawing.Imaging;
public void ConvertWordToImage(string wordPath, string imagePath)
{
// 加载Word文档
Document doc = new Document(wordPath);
// 遍历文档的每一页
for (int pageIndex = 0; pageIndex < doc.PageCount; pageIndex++)
{
// 将每一页保存为图片格式
string pageImagePath = $"{imagePath}_{pageIndex + 1}.jpg";
doc.Save(pageImagePath, SaveFormat.Jpeg);
}
}
public void ConvertWordToPDF(string wordPath, string pdfPath)
{
// 加载Word文档
Document doc = new Document(wordPath);
// 将文档保存为PDF格式
doc.Save(pdfPath, SaveFormat.Pdf);
}
```
使用以上代码示例,可以将指定路径的Word文档转换为图片或PDF格式。可通过`ConvertWordToImage`方法将Word转为图片,每一页生成一张图片;通过`ConvertWordToPDF`方法可将Word转为PDF。在使用代码前,需将Aspose.Words的相关库导入到项目中,并在代码中引用相应的命名空间。
需要注意的是,Aspose.Words是一个商业类库,提供了强大的Word处理功能,但其使用可能需要支付费用。另外,还有其他一些开源的库,如Spire.Doc等,也提供了类似的功能,你也可以选择适合自己项目的组件来实现Word转换为图片或PDF。
asp图片生成pdf
ASP 不是生成 PDF 的最佳工具,但你可以使用一些第三方库来生成 PDF。其中一个流行的库是 iTextSharp。以下是一个简单的 ASP.NET 示例,演示如何使用 iTextSharp 生成 PDF:
```csharp
using iTextSharp.text;
using iTextSharp.text.pdf;
protected void GeneratePDF_Click(object sender, EventArgs e)
{
// 创建一个新的 PDF 文档
Document document = new Document(PageSize.A4, 50, 50, 25, 25);
PdfWriter writer = PdfWriter.GetInstance(document, new FileStream("C:/example.pdf", FileMode.Create));
document.Open();
// 添加一张图片到 PDF 文档
iTextSharp.text.Image image = iTextSharp.text.Image.GetInstance(Server.MapPath("~/images/example.jpg"));
image.ScaleToFit(document.PageSize.Width - 50, document.PageSize.Height - 50);
document.Add(image);
// 关闭 PDF 文档
document.Close();
}
```
在此示例中,我们创建了一个新的 PDF 文档,将一张图片添加到其中,然后将其保存到磁盘上的一个文件中。你可以通过调整代码来适应你的需求,例如改变页面大小、位置等。
相关推荐
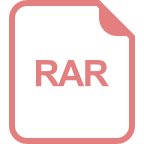
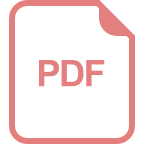
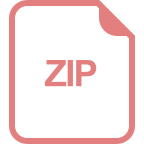












