记账本android studio实现
时间: 2023-09-15 20:16:35 浏览: 52
1. 首先,在Android Studio中创建一个新的项目。
2. 在项目的build.gradle文件中添加以下依赖项:
```
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
```
3. 创建一个RecyclerView,用于显示记账记录。在activity_main.xml文件中添加以下代码:
```
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
</android.support.constraint.ConstraintLayout>
```
4. 创建一个记账记录的数据类,包括金额、时间、类型等信息。
```
public class Record {
private int id;
private int amount;
private String time;
private String type;
public Record(int id, int amount, String time, String type) {
this.id = id;
this.amount = amount;
this.time = time;
this.type = type;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getAmount() {
return amount;
}
public void setAmount(int amount) {
this.amount = amount;
}
public String getTime() {
return time;
}
public void setTime(String time) {
this.time = time;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
}
```
5. 创建一个RecyclerView的适配器类,用于将记账记录数据显示在RecyclerView中。
```
public class RecordAdapter extends RecyclerView.Adapter<RecordAdapter.ViewHolder> {
private List<Record> mRecordList;
static class ViewHolder extends RecyclerView.ViewHolder {
TextView amountTextView;
TextView timeTextView;
TextView typeTextView;
public ViewHolder(View view) {
super(view);
amountTextView = view.findViewById(R.id.amountTextView);
timeTextView = view.findViewById(R.id.timeTextView);
typeTextView = view.findViewById(R.id.typeTextView);
}
}
public RecordAdapter(List<Record> recordList) {
mRecordList = recordList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.record_item, parent, false);
ViewHolder holder = new ViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
Record record = mRecordList.get(position);
holder.amountTextView.setText(String.valueOf(record.getAmount()));
holder.timeTextView.setText(record.getTime());
holder.typeTextView.setText(record.getType());
}
@Override
public int getItemCount() {
return mRecordList.size();
}
}
```
6. 创建一个记账记录的数据库类,用于存储和读取记账记录数据。
```
public class RecordDatabaseHelper extends SQLiteOpenHelper {
public static final String CREATE_RECORD = "create table Record ("
+ "id integer primary key autoincrement, "
+ "amount integer, "
+ "time text, "
+ "type text)";
public RecordDatabaseHelper(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, factory, version);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_RECORD);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("drop table if exists Record");
onCreate(db);
}
public void addRecord(Record record) {
SQLiteDatabase db = getWritableDatabase();
ContentValues values = new ContentValues();
values.put("amount", record.getAmount());
values.put("time", record.getTime());
values.put("type", record.getType());
db.insert("Record", null, values);
db.close();
}
public void deleteRecord(int id) {
SQLiteDatabase db = getWritableDatabase();
db.delete("Record", "id=?", new String[]{String.valueOf(id)});
db.close();
}
public List<Record> getAllRecords() {
List<Record> recordList = new ArrayList<>();
SQLiteDatabase db = getReadableDatabase();
Cursor cursor = db.query("Record", null, null, null, null, null, null);
if (cursor.moveToFirst()) {
do {
int id = cursor.getInt(cursor.getColumnIndex("id"));
int amount = cursor.getInt(cursor.getColumnIndex("amount"));
String time = cursor.getString(cursor.getColumnIndex("time"));
String type = cursor.getString(cursor.getColumnIndex("type"));
Record record = new Record(id, amount, time, type);
recordList.add(record);
} while (cursor.moveToNext());
}
cursor.close();
db.close();
return recordList;
}
}
```
7. 创建一个MainActivity类,用于管理记账记录的添加、删除和显示。在onCreate方法中,实例化RecyclerView和RecordAdapter,并将适配器设置给RecyclerView。在添加按钮的点击事件中,获取用户输入的金额、时间和类型,将其保存到数据库中,并更新RecyclerView的显示。在RecyclerView的item中,添加删除按钮的点击事件,将记录从数据库中删除,并更新RecyclerView的显示。
```
public class MainActivity extends AppCompatActivity {
private List<Record> mRecordList = new ArrayList<>();
private RecordAdapter mRecordAdapter;
private RecordDatabaseHelper mDatabaseHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDatabaseHelper = new RecordDatabaseHelper(this, "Record.db", null, 1);
mRecordList = mDatabaseHelper.getAllRecords();
RecyclerView recyclerView = findViewById(R.id.recyclerView);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
mRecordAdapter = new RecordAdapter(mRecordList);
recyclerView.setAdapter(mRecordAdapter);
Button addButton = findViewById(R.id.addButton);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText amountEditText = findViewById(R.id.amountEditText);
EditText timeEditText = findViewById(R.id.timeEditText);
EditText typeEditText = findViewById(R.id.typeEditText);
int amount = Integer.parseInt(amountEditText.getText().toString());
String time = timeEditText.getText().toString();
String type = typeEditText.getText().toString();
Record record = new Record(0, amount, time, type);
mDatabaseHelper.addRecord(record);
mRecordList.clear();
mRecordList.addAll(mDatabaseHelper.getAllRecords());
mRecordAdapter.notifyDataSetChanged();
amountEditText.setText("");
timeEditText.setText("");
typeEditText.setText("");
}
});
}
public void deleteRecord(View view) {
int position = (int) view.getTag();
Record record = mRecordList.get(position);
mDatabaseHelper.deleteRecord(record.getId());
mRecordList.remove(position);
mRecordAdapter.notifyItemRemoved(position);
}
}
```
相关推荐
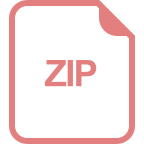














