但是我这样没有获取到File imgfile=new File("坤1.jpg");
时间: 2024-03-21 13:42:31 浏览: 11
如果您的servlet与图片文件"坤1.jpg"在同一目录下,那么可以使用以下代码来获取图片文件的绝对路径:
```java
String imgPath = getServletContext().getRealPath("坤1.jpg");
File imgFile = new File(imgPath);
```
其中,`getServletContext().getRealPath("坤1.jpg")`用于获取图片文件的绝对路径,然后通过`File`类来创建一个`File`对象。这样就可以使用`imgFile`对象来操作图片文件了。
请注意,如果您正在开发一个Web应用程序并部署到Tomcat等Web容器中,那么获取文件的绝对路径时应使用`getServletContext().getRealPath()`方法,而不是使用相对路径。因为在不同的环境中,相对路径可能会有所不同,而绝对路径则更加可靠。
相关问题
// 引入OpenCV库 #include "D:/opencv/4.7.0/opencv.js" // 创建一个新的AE合成 var comp = app.project.items.addComp("OpenCV Example", 1920, 1080, 1, 10, 30); // 加载待识别的图片 var img = new File("C:/Users/Administrator/Pictures/Screenshots/屏幕截图(4).png"); alert(img.fsName) // 使用OpenCV加载图片 var mat = cv.imread(img.fsName); // 对图片进行处理,例如转换为灰度图像 cv.cvtColor(mat, mat, cv.COLOR_RGB2GRAY); // 显示处理后的图片 var viewer = comp.layer("视图"); var viewerSource = viewer.source; viewerSource.width = mat.cols; viewerSource.height = mat.rows; viewerSource.pixelAspect = 1; var pixels = viewerSource.sourceRect.width * viewerSource.sourceRect.height; viewerSource.setProxyForMissingFrames(true); viewerSource.setProxyToNone(); viewerSource.numFrames = pixels; viewerSource.duration = 1 / viewerSource.frameRate; for (var i = 0; i < pixels; i++) { var data = mat.data; var pixel = 0; viewerSource.setFrameDuration(i, viewerSource.duration); viewerSource.setFrameRenderingQuality(i, true); viewerSource.setFrameSurfaceForFrame(i, true); viewerSource.setFrameSurface(i, surface); for (var j = 0; j < viewerSource.height; j++) { for (var k = 0; k < viewerSource.width; k++) { var value = data[pixel++]; viewerSource.setPixel(k, j, [value, value, value]); } } } // 保存处理后的图片 cv.imwrite("C:/Users/Administrator/Pictures/Screenshots/processed_image.jpg", mat);帮我优化这段代码
1. 将OpenCV库引入放在代码最后,这样可以避免在执行到引入代码时出现错误导致整个程序崩溃的情况。
2. 在加载图片之前,最好先判断图片文件是否存在,避免出现文件不存在的错误。
3. 在处理图片时,可以使用OpenCV提供的函数代替嵌套循环,可以提高代码效率。
4. 在设置像素值时,可以使用OpenCV提供的at函数代替data数组,可以提高代码可读性和效率。
5. 在循环设置像素值时,可以使用setPixels函数代替setPixel函数,可以提高代码效率。
改进后的代码如下:
```javascript
// 创建一个新的AE合成
var comp = app.project.items.addComp("OpenCV Example", 1920, 1080, 1, 10, 30);
// 加载待识别的图片
var imgFilePath = "C:/Users/Administrator/Pictures/Screenshots/屏幕截图(4).png";
var imgFile = new File(imgFilePath);
if (!imgFile.exists) {
alert("图片文件不存在!");
exit();
}
// 使用OpenCV加载图片
var mat = cv.imread(imgFilePath);
// 对图片进行处理,例如转换为灰度图像
cv.cvtColor(mat, mat, cv.COLOR_RGB2GRAY);
// 显示处理后的图片
var viewer = comp.layer("视图");
var viewerSource = viewer.source;
viewerSource.width = mat.cols;
viewerSource.height = mat.rows;
viewerSource.pixelAspect = 1;
var pixels = viewerSource.sourceRect.width * viewerSource.sourceRect.height;
viewerSource.setProxyForMissingFrames(true);
viewerSource.setProxyToNone();
viewerSource.numFrames = pixels;
viewerSource.duration = 1 / viewerSource.frameRate;
for (var i = 0; i < pixels; i++) {
var pixel = 0;
viewerSource.setFrameDuration(i, viewerSource.duration);
viewerSource.setFrameRenderingQuality(i, true);
viewerSource.setFrameSurfaceForFrame(i, true);
viewerSource.setFrameSurface(i, surface);
var row = Math.floor(i / viewerSource.width);
var col = i % viewerSource.width;
var value = mat.at(row, col);
viewerSource.setPixels(col, row, 1, 1, [value, value, value]);
}
// 保存处理后的图片
cv.imwrite("C:/Users/Administrator/Pictures/Screenshots/processed_image.jpg", mat);
// 引入OpenCV库
#include "D:/opencv/4.7.0/opencv.js"
```
根据绝对路径绑定适配器并打开图片
在Android中,应用程序无法直接访问文件系统中的绝对路径,因此您需要将绝对路径转换为Android的URI格式,然后使用该URI加载图像。以下是将绝对路径转换为URI并加载图像的示例代码:
```java
File imgFile = new File("/storage/emulated/0/Pictures/image.jpg");
if(imgFile.exists()){
// 将绝对路径转换为URI
Uri uri = Uri.fromFile(imgFile);
// 加载图像到ImageView中
ImageView imageView = findViewById(R.id.imageView);
imageView.setImageURI(uri);
}
```
在这个示例中,我们首先创建一个File对象来表示我们要加载的图像文件。然后,我们检查文件是否存在,并将其绝对路径转换为Android的URI格式。最后,我们将URI传递给ImageView,以便将图像加载到其中。
如果您想要在RecyclerView或ListView中显示多个图像,则需要创建一个适配器,并使用该适配器将图像绑定到列表项中。以下是一个简单的示例,演示如何使用绝对路径创建适配器并将其绑定到RecyclerView中:
```java
public class ImageAdapter extends RecyclerView.Adapter<ImageAdapter.ImageViewHolder> {
private List<String> mImagePaths;
public ImageAdapter(List<String> imagePaths) {
mImagePaths = imagePaths;
}
@NonNull
@Override
public ImageViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.list_item_image, parent, false);
return new ImageViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ImageViewHolder holder, int position) {
String imagePath = mImagePaths.get(position);
File imgFile = new File(imagePath);
if (imgFile.exists()) {
Uri uri = Uri.fromFile(imgFile);
holder.mImageView.setImageURI(uri);
}
}
@Override
public int getItemCount() {
return mImagePaths.size();
}
public static class ImageViewHolder extends RecyclerView.ViewHolder {
private ImageView mImageView;
public ImageViewHolder(@NonNull View itemView) {
super(itemView);
mImageView = itemView.findViewById(R.id.imageView);
}
}
}
```
在这个示例中,我们创建了一个适配器类ImageAdapter,该适配器类从一个字符串列表中获取图像的绝对路径,并将其绑定到RecyclerView中。在适配器的onBindViewHolder方法中,我们将每个图像的绝对路径转换为URI,并将其设置为ImageView的图像源。最后,我们在RecyclerView的布局文件中使用ImageView来显示图像。
相关推荐
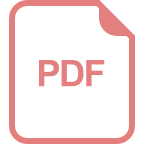
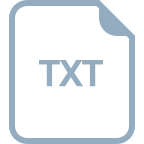
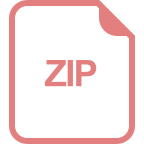











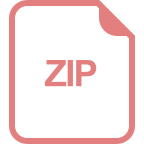
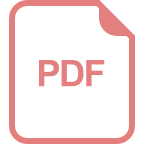