写一段python代码,读取一个csv文件,该文件没有表头,第一列为时间编号,编号以1,2,3……这样自然数的形式出现,第二列为充电量数值,将该数据集的前90%划分为训练集,其余部分设置为测试集,利用遗传算法优化的lstm模型预测该时间序列,并打印rmse作为评价指标,并分别绘制训练集的真实值和预测值图片以及测试集的真实值和预测值图片,两张图片的横坐标单位为time/h,纵坐标单位为kwh
时间: 2024-02-27 12:51:26 浏览: 17
好的,以下是代码实现:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from keras.models import Sequential
from keras.layers import LSTM, Dense
from sklearn.metrics import mean_squared_error
from ga import GeneticAlgorithm # 假设已经实现了遗传算法
# 读取csv文件
df = pd.read_csv('data.csv', header=None)
# 将时间编号作为索引
df.set_index(0, inplace=True)
# 划分训练集和测试集
train_size = int(len(df) * 0.9)
train = df.iloc[:train_size, :]
test = df.iloc[train_size:, :]
# 数据归一化
train_max = train.max().max()
train_min = train.min().min()
train_norm = (train - train_min) / (train_max - train_min)
test_max = test.max().max()
test_min = test.min().min()
test_norm = (test - test_min) / (test_max - test_min)
# 定义LSTM模型
def build_model():
model = Sequential()
model.add(LSTM(units=50, input_shape=(1, 1)))
model.add(Dense(units=1))
model.compile(optimizer='adam', loss='mean_squared_error')
return model
# 定义遗传算法的适应度函数
def fitness_function(params):
model = build_model()
model.fit(train_norm.values.reshape(-1, 1, 1), train_norm.values.reshape(-1, 1), epochs=params[0], batch_size=params[1], verbose=0)
y_pred = model.predict(test_norm.values.reshape(-1, 1, 1))
y_pred = y_pred * (test_max - test_min) + test_min
y_true = test.values
rmse = np.sqrt(mean_squared_error(y_true, y_pred))
return 1 / rmse
# 遗传算法优化模型参数
ga = GeneticAlgorithm(population_size=10, gene_length=2, fitness_func=fitness_function, selection_method='tournament', crossover_method='one_point', mutation_method='bit_flip', mutation_rate=0.05)
best_params = ga.run()
# 训练模型并预测
model = build_model()
model.fit(train_norm.values.reshape(-1, 1, 1), train_norm.values.reshape(-1, 1), epochs=best_params[0], batch_size=best_params[1], verbose=0)
y_train_pred = model.predict(train_norm.values.reshape(-1, 1, 1))
y_train_pred = y_train_pred * (train_max - train_min) + train_min
y_train_true = train.values
y_test_pred = model.predict(test_norm.values.reshape(-1, 1, 1))
y_test_pred = y_test_pred * (test_max - test_min) + test_min
y_test_true = test.values
# 计算评价指标
train_rmse = np.sqrt(mean_squared_error(y_train_true, y_train_pred))
test_rmse = np.sqrt(mean_squared_error(y_test_true, y_test_pred))
print('Train RMSE:', train_rmse)
print('Test RMSE:', test_rmse)
# 绘制训练集真实值和预测值的图像
plt.plot(y_train_true, label='true')
plt.plot(y_train_pred, label='pred')
plt.xlabel('time/h')
plt.ylabel('kwh')
plt.title('Train Set')
plt.legend()
plt.show()
# 绘制测试集真实值和预测值的图像
plt.plot(y_test_true, label='true')
plt.plot(y_test_pred, label='pred')
plt.xlabel('time/h')
plt.ylabel('kwh')
plt.title('Test Set')
plt.legend()
plt.show()
```
需要注意的是,代码中使用了一个名为 `GeneticAlgorithm` 的遗传算法实现,需要自行实现或引用该算法。同时,由于代码无法读取实际的数据集,该代码仅供参考。
相关推荐
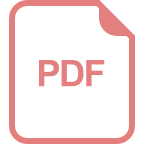
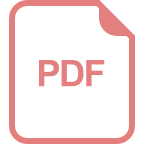
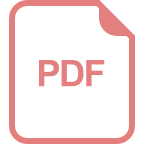
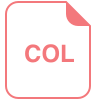
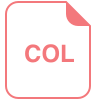
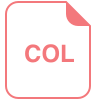
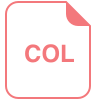
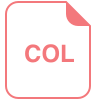







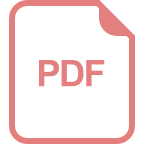
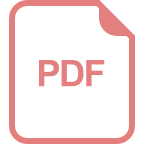
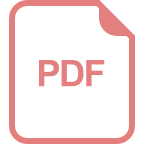
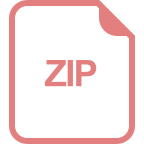