#include<iostream> #include<cstring> using namespace std; class MyclassA{ public: MyclassA(char *s) { strcpy(str,s); } void print() { cout<<str<<endl; } protected: char str[100]; }; class MyclassB{ public: MyclassB(char *s) { strcpy(str,s); } void print() { cout<<str<<endl; } protected: char str[100]; }; class MyclassC:public MyclassA,public MyclassB{ public: MyclassC(char *s1,char *s2):MyclassA(s1),MyclassB(s2) { } void splicing() { strcpy(str,MyclassA::str); //ERROR**********found********** strcat(str,MyclassB::str); } void print() { cout<<str<<endl; } private: char str[200]; }; int main(void) { MyclassC test("stringA","stringB"); //ERROR**********found********** test.print(); // 输出stringA //ERROR**********found********** test.print(); // 输出stringB test.splicing(); test.print(); // 输出stringAstringB system("pause"); return 0; }
时间: 2023-07-23 13:55:08 浏览: 228
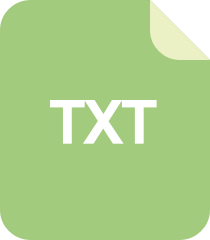
#include_iostream.h_

这段代码是一个简单的多继承例子,定义了三个类 MyclassA、MyclassB 和 MyclassC,其中 MyclassA 和 MyclassB 都有一个 char 类型的成员变量 str 和一个 print() 函数用于输出该变量的值,而 MyclassC 继承了 MyclassA 和 MyclassB,定义了一个新的成员变量 str 和一个 splicing() 函数用于将 MyclassA 和 MyclassB 的 str 变量拼接起来,并且重载了 MyclassA 和 MyclassB 的 print() 函数。
在 main() 函数中,创建了一个 MyclassC 的实例 test,传入了两个参数分别为 "stringA" 和 "stringB",然后分别调用了两次 test.print() 函数,第一次输出 "stringA",第二次输出 "stringB",最后调用了 test.splicing() 函数将 MyclassA 和 MyclassB 的 str 变量拼接起来,并输出拼接后的结果 "stringAstringB"。
阅读全文
相关推荐
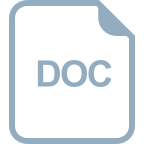
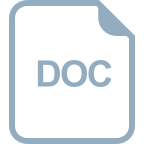
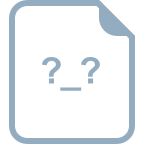

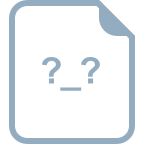
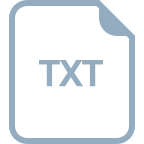
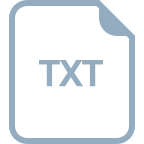
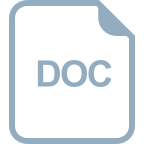

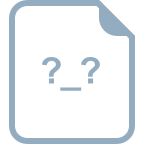
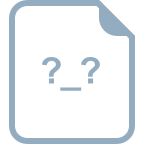
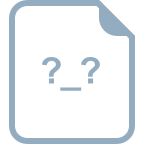
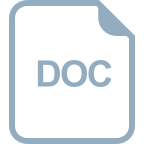
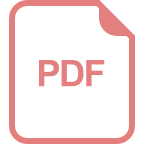


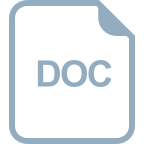