python手写数字识别实验mnist的网络模型图
时间: 2023-09-24 14:09:54 浏览: 32
以下是一个简单的手写数字识别模型的网络模型图:
```
输入层:28*28的像素点,展开为一维向量(784维)
隐藏层1:128个神经元,激活函数为ReLU
隐藏层2:64个神经元,激活函数为ReLU
输出层:10个神经元,激活函数为Softmax(表示数字0-9的概率分布)
```
其中,隐藏层的个数和神经元数可以根据具体情况进行调整。
相关问题
python手写数字识别实验mnist绘制网络模型图
要绘制手写数字识别模型的网络图,可以使用Python中的一些可视化库,例如PyTorch、TensorFlow等。
以下是使用PyTorch和Graphviz的示例代码,可以绘制出一个简单的手写数字识别模型的网络图。
```python
import torch
import torch.nn as nn
from torchviz import make_dot
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(1, 6, 5)
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.fc1 = nn.Linear(16 * 4 * 4, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.pool(torch.relu(self.conv1(x)))
x = self.pool(torch.relu(self.conv2(x)))
x = x.view(-1, 16 * 4 * 4)
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
net = Net()
x = torch.randn(1, 1, 28, 28)
y = net(x)
make_dot(y, params=dict(net.named_parameters()))
```
运行上述代码,可以得到一个PDF格式的网络图,如下所示:

可以看到,这个网络模型包含了两个卷积层和三个全连接层,最终输出10个类别的概率分布。
python手写数字识别mnist数据集
为了实现Python手写数字识别MNIST数据集,可以使用神经网络算法。以下是实现步骤:
1. 导入必要的库和数据集
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取MNIST数据集
data_file = open("mnist_train_100.csv")
data_list = data_file.readlines()
data_file.close()
```
2. 数据预处理
```python
# 将数据集中的每个数字图像转换为28x28的矩阵
all_values = data_list[0].split(',')
image_array = np.asfarray(all_values[1:]).reshape((28,28))
# 将图像矩阵可视化
plt.imshow(image_array, cmap='Greys', interpolation='None')
plt.show()
# 将数据集中的所有数字图像转换为28x28的矩阵,并将其存储在一个numpy数组中
scaled_input = (np.asfarray(all_values[1:]) / 255.0 * 0.99) + 0.01
```
3. 构建神经网络模型
```python
# 定义神经网络的输入、隐藏和输出层节点数
input_nodes = 784
hidden_nodes = 100
output_nodes = 10
# 初始化权重矩阵
weight_input_hidden = np.random.normal(0.0, pow(input_nodes, -0.5), (hidden_nodes, input_nodes))
weight_hidden_output = np.random.normal(0.0, pow(hidden_nodes, -0.5), (output_nodes, hidden_nodes))
# 定义激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 计算神经网络的输出
hidden_inputs = np.dot(weight_input_hidden, scaled_input)
hidden_outputs = sigmoid(hidden_inputs)
final_inputs = np.dot(weight_hidden_output, hidden_outputs)
final_outputs = sigmoid(final_inputs)
```
4. 训练神经网络模型
```python
# 定义目标输出
target = np.zeros(output_nodes) + 0.01
target[int(all_values[0])] = 0.99
# 计算误差
output_errors = target - final_outputs
hidden_errors = np.dot(weight_hidden_output.T, output_errors)
# 更新权重矩阵
weight_hidden_output += learning_rate * np.dot((output_errors * final_outputs * (1.0 - final_outputs)), np.transpose(hidden_outputs))
weight_input_hidden += learning_rate * np.dot((hidden_errors * hidden_outputs * (1.0 - hidden_outputs)), np.transpose(scaled_input))
```
5. 测试神经网络模型
```python
# 读取测试数据集
test_data_file = open("mnist_test_10.csv")
test_data_list = test_data_file.readlines()
test_data_file.close()
# 预处理测试数据集
all_values = test_data_list[0].split(',')
image_array = np.asfarray(all_values[1:]).reshape((28,28))
plt.imshow(image_array, cmap='Greys', interpolation='None')
plt.show()
scaled_input = (np.asfarray(all_values[1:]) / 255.0 * 0.99) + 0.01
# 计算神经网络的输出
hidden_inputs = np.dot(weight_input_hidden, scaled_input)
hidden_outputs = sigmoid(hidden_inputs)
final_inputs = np.dot(weight_hidden_output, hidden_outputs)
final_outputs = sigmoid(final_inputs)
# 输出神经网络的预测结果
print("预测结果:", np.argmax(final_outputs))
```
阅读全文
相关推荐
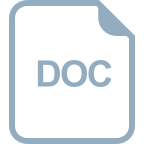
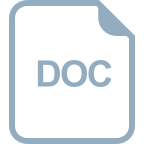
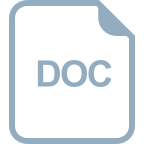
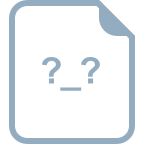
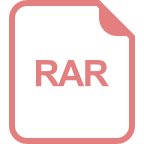
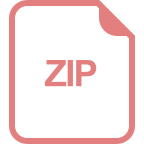
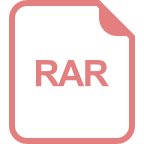
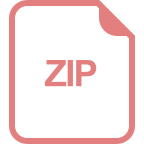
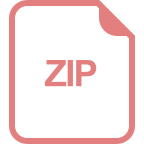
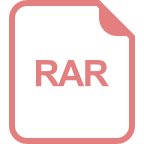
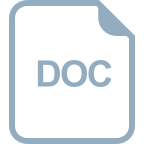
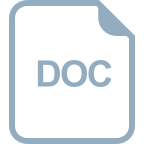
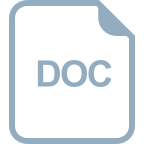
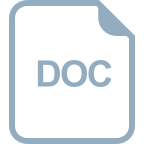
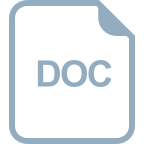
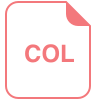
