使用vue编写一个弹出的输入框
时间: 2023-06-19 15:05:18 浏览: 110
可以使用Vue.js的组件来编写一个弹出的输入框。
首先,创建一个新的Vue组件,例如InputBox.vue:
```html
<template>
<div class="input-box" v-if="show">
<div class="overlay"></div>
<div class="dialog">
<label>{{label}}</label>
<input type="text" v-model="value">
<div class="buttons">
<button @click="cancel">取消</button>
<button @click="confirm">确定</button>
</div>
</div>
</div>
</template>
<script>
export default {
props: {
label: {
type: String,
required: true
},
value: {
type: String,
default: ''
},
show: {
type: Boolean,
default: false
}
},
methods: {
cancel() {
this.$emit('cancel');
},
confirm() {
this.$emit('confirm', this.value);
}
}
}
</script>
<style>
.input-box {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
.overlay {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5);
}
.dialog {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 6px rgba(0, 0, 0, 0.3);
}
.dialog label {
display: block;
margin-bottom: 10px;
}
.dialog input[type="text"] {
width: 100%;
border: 1px solid #ccc;
border-radius: 3px;
padding: 5px;
margin-bottom: 10px;
}
.dialog button {
margin-right: 10px;
}
</style>
```
在这个组件中,我们使用了一个v-if指令来控制组件的显示和隐藏。当show属性为true时,组件会显示在页面上。组件中包含一个遮罩层(overlay),一个对话框(dialog),一个标签(label),一个文本输入框(input),和两个按钮(取消和确定)。
在组件中定义了三个props:label、value和show。label表示输入框的标签,value表示输入框的初始值,show表示是否显示输入框。
在组件中定义了两个方法:cancel和confirm。当用户点击取消按钮时,组件会触发cancel方法并通过$emit方法向父组件传递cancel事件。当用户点击确定按钮时,组件会触发confirm方法并通过$emit方法向父组件传递confirm事件和输入框的值。
在父组件中,可以使用v-model指令来绑定输入框的值,并使用v-on指令来监听cancel和confirm事件。例如:
```html
<template>
<div>
<button @click="showInputBox = true">打开输入框</button>
<input-box label="请输入内容" v-model="inputValue" :show="showInputBox" @cancel="showInputBox = false" @confirm="onInputConfirmed"></input-box>
</div>
</template>
<script>
import InputBox from './InputBox.vue';
export default {
components: {
InputBox
},
data() {
return {
showInputBox: false,
inputValue: ''
}
},
methods: {
onInputConfirmed(value) {
console.log('输入的内容是:', value);
this.showInputBox = false;
}
}
}
</script>
```
在这个父组件中,我们使用了一个按钮来触发显示输入框。当用户点击按钮时,showInputBox属性会被设置为true,从而显示输入框。输入框的值会通过v-model指令绑定到inputValue属性上。
当用户点击取消按钮时,输入框会关闭并且showInputBox属性会被设置为false。当用户点击确定按钮时,输入框会关闭并且会调用onInputConfirmed方法来处理输入框的值。在这个例子中,我们只是简单地将输入框的值打印到控制台上,但是您可以根据需要在这里执行任何逻辑。
阅读全文
相关推荐
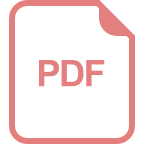
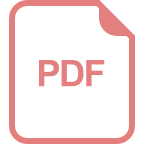



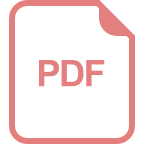
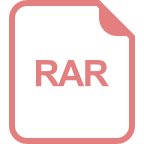
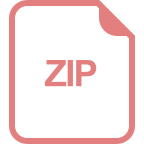
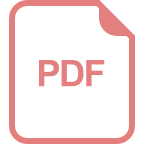
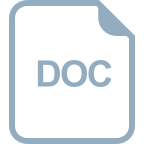
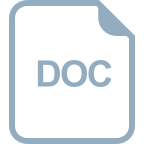
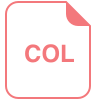





