import numpy as np import tensorflow_datasets as tfds import tensorflow as tf tfds.disable_progress_bar() import matplotlib.pyplot as plt def plot_graphs(history, metric): plt.plot(history.history[metric]) plt.plot(history.history['val_'+metric], '') plt.xlabel("Epochs") plt.ylabel(metric) plt.legend([metric, 'val_'+metric]) dataset, info = tfds.load('imdb_reviews', with_info=True, as_supervised=True) train_dataset, test_dataset = dataset['train'], dataset['test'] train_dataset.element_spec for example, label in train_dataset.take(1): print('text: ', example.numpy()) print('label: ', label.numpy()) BUFFER_SIZE = 10000 BATCH_SIZE = 64 train_dataset = train_dataset.shuffle(BUFFER_SIZE).batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE) test_dataset = test_dataset.batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE) for example, label in train_dataset.take(1): print('texts: ', example.numpy()[:3]) print() print('labels: ', label.numpy()[:3]) VOCAB_SIZE = 1000 encoder = tf.keras.layers.TextVectorization( max_tokens=VOCAB_SIZE) encoder.adapt(train_dataset.map(lambda text, label: text)) vocab = np.array(encoder.get_vocabulary()) vocab[:20] encoded_example = encoder(example)[:3].numpy() encoded_example for n in range(3): print("Original: ", example[n].numpy()) print("Round-trip: ", " ".join(vocab[encoded_example[n]])) print()解释一下这些代码。
时间: 2023-11-27 07:49:35 浏览: 39
这段代码是使用TensorFlow和TensorFlow Datasets加载IMDB电影评论数据集,然后对数据集进行预处理和向量化,以便进行情感分析的训练。下面是代码的详细解释:
1. 导入必要的库:
```
import numpy as np
import tensorflow_datasets as tfds
import tensorflow as tf
import matplotlib.pyplot as plt
```
2. 禁用进度条,以免在输出过程中出现过多的信息:
```
tfds.disable_progress_bar()
```
3. 加载IMDB评论数据集并获取有关数据集的信息:
```
dataset, info = tfds.load('imdb_reviews', with_info=True, as_supervised=True)
train_dataset, test_dataset = dataset['train'], dataset['test']
```
4. 查看数据集中的一个示例,并输出文本和标签:
```
for example, label in train_dataset.take(1):
print('text: ', example.numpy())
print('label: ', label.numpy())
```
5. 定义缓冲区大小和批次大小,并对训练和测试数据集进行批处理和预取:
```
BUFFER_SIZE = 10000
BATCH_SIZE = 64
train_dataset = train_dataset.shuffle(BUFFER_SIZE).batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE)
test_dataset = test_dataset.batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE)
```
6. 查看训练数据集中的前三个示例的文本和标签:
```
for example, label in train_dataset.take(1):
print('texts: ', example.numpy()[:3])
print()
print('labels: ', label.numpy()[:3])
```
7. 定义词汇表大小和文本向量化层,然后使用`adapt`方法对训练数据集进行适应:
```
VOCAB_SIZE = 1000
encoder = tf.keras.layers.TextVectorization(max_tokens=VOCAB_SIZE)
encoder.adapt(train_dataset.map(lambda text, label: text))
```
8. 获取词汇表并输出前20个词汇:
```
vocab = np.array(encoder.get_vocabulary())
vocab[:20]
```
9. 对一个示例进行编码,并输出编码结果:
```
encoded_example = encoder(example)[:3].numpy()
encoded_example
```
10. 对编码后的示例进行反向转换并输出结果:
```
for n in range(3):
print("Original: ", example[n].numpy())
print("Round-trip: ", " ".join(vocab[encoded_example[n]]))
print()
```
该代码段中的主要任务是将IMDB评论数据集加载到TensorFlow中,并准备进行情感分析训练。它包含了对数据的处理、向量化和预处理等步骤,是进行自然语言处理任务的常见流程。
相关推荐
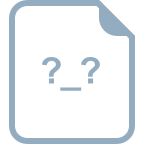
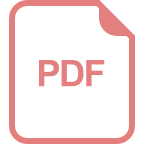
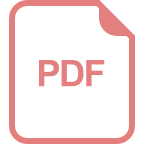















