用c语言写一个串口通信协议
时间: 2023-05-14 22:04:25 浏览: 119
好的,以下是一个简单的串口通信协议的 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define BAUDRATE B9600
#define MODEMDEVICE "/dev/ttyS0"
#define _POSIX_SOURCE 1
int main()
{
int fd, c, res;
struct termios oldtio, newtio;
char buf[255];
fd = open(MODEMDEVICE, O_RDWR | O_NOCTTY);
if (fd < 0)
{
perror(MODEMDEVICE);
exit(1);
}
tcgetattr(fd, &oldtio);
bzero(&newtio, sizeof(newtio));
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = IGNPAR;
newtio.c_oflag = 0;
newtio.c_cc[VTIME] = 0;
newtio.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &newtio);
while (1)
{
res = read(fd, buf, 255);
if (res > 0)
{
buf[res] = 0;
printf("Received: %s\n", buf);
}
c = getchar();
if (c == 'q' || c == 'Q')
break;
write(fd, &c, 1);
}
tcsetattr(fd, TCSANOW, &oldtio);
close(fd);
return 0;
}
```
这个程序使用了 Linux 的串口设备文件 `/dev/ttyS0`,并且使用了标准的 9600 波特率。它会不断地读取串口数据,并将其打印出来,同时也可以从标准输入中读取字符并发送到串口。当输入字符为 'q' 或 'Q' 时,程序会退出。
相关推荐
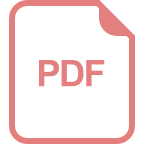
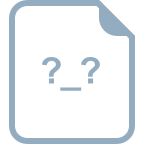














