springboot cxf调用webservice
时间: 2023-05-31 10:18:26 浏览: 317
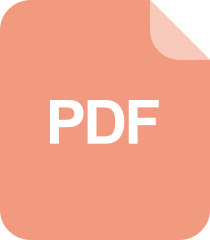
springboot整合cxf发布webservice以及调用的方法
### 回答1:
Spring Boot集成CXF框架可以方便地调用Web服务。以下是使用Spring Boot和CXF调用Web服务的步骤:
1. 在pom.xml文件中添加CXF依赖:
```
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.3.6</version>
</dependency>
```
2. 创建一个接口来定义Web服务的操作:
```
@WebService
public interface MyWebService {
@WebMethod
String sayHello(String name);
}
```
3. 创建一个实现类来实现接口中定义的操作:
```
@Service
@WebService(endpointInterface = "com.example.MyWebService")
public class MyWebServiceImpl implements MyWebService {
@Override
public String sayHello(String name) {
return "Hello " + name + "!";
}
}
```
4. 在应用程序的配置文件中添加CXF配置:
```
cxf:
servlet:
path: /services/*
jaxws:
properties:
javax.xml.ws.soap.http.soapaction.use: "false"
```
5. 在控制器中注入Web服务并调用它:
```
@RestController
public class MyController {
@Autowired
private MyWebService myWebService;
@GetMapping("/hello/{name}")
public String sayHello(@PathVariable String name) {
return myWebService.sayHello(name);
}
}
```
6. 启动应用程序并访问Web服务:
```
http://localhost:8080/services/MyWebServiceImpl?wsdl
```
以上就是使用Spring Boot和CXF调用Web服务的步骤。
### 回答2:
Spring Boot是一个使用习惯优秀的Web应用开发框架,它可以帮助我们快速构建应用,提高开发效率。而CXF是一个开源的WebService框架,它提供了一系列的API和工具来帮助开发人员可以很轻易地实现一个基于SOAP的Web服务。
在Spring Boot中调用CXF框架中的WebService,需要进行以下步骤:
1. 添加依赖
在pom.xml中添加CXF和Spring Boot Web依赖:
```
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.3.1</version>
</dependency>
</dependencies>
```
2. 编写WebService客户端类
编写一个类来调用CXF产生的webservice服务,其中包括Endpoint指向和需要调用的方法等信息。
```
@Service
public class WebServiceClient {
@Autowired
private JaxWsProxyFactoryBean jaxWsProxyFactoryBean;
private HelloPortType helloPortType;
public String sayHello(final String name) {
initPortType();
return helloPortType.sayHello(name);
}
private void initPortType() {
if (helloPortType == null) {
jaxWsProxyFactoryBean.setServiceClass(HelloPortType.class);
jaxWsProxyFactoryBean.setAddress("http://localhost:8080/hello");
helloPortType = (HelloPortType) jaxWsProxyFactoryBean.create();
}
}
}
```
3. 编写WebService
通过CXF创建web服务,并实现接口提供服务,返回接口需要的数据。
```
@javax.jws.WebService(serviceName = "HelloService", portName = "HelloPort", targetNamespace = "http://www.example.org/HelloService/", endpointInterface = "org.example.service.HelloPortType")
public class HelloPortTypeImpl implements HelloPortType {
private final static Logger LOGGER = LoggerFactory.getLogger(HelloPortTypeImpl.class);
@Resource
private WebServiceContext webServiceContext;
@Override
public String sayHello(final String name) {
final MessageContext mc = webServiceContext.getMessageContext();
final HttpServletRequest req = (HttpServletRequest) mc.get(MessageContext.SERVLET_REQUEST);
LOGGER.info("服务SayHello calling, name: {}, IP addr:{}, sessionId: {}", name, req.getRemoteAddr(), req.getSession().getId());
return String.format("Hello, %s!", name);
}
}
```
4. 进行测试
在控制器中注入WebService客户端类,并进行测试。
```
@RestController
@RequestMapping("/test")
public class TestController {
@Autowired
private WebServiceClient webServiceClient;
@GetMapping("/sayHello")
public String sayHello(@RequestParam(value = "name") final String name) {
return webServiceClient.sayHello(name);
}
}
```
总的来说,Spring Boot和CXF框架结合起来使用,可以很方便地调用WebService,提供服务的代码也可以很容易地进行编写。
### 回答3:
SpringBoot是一个非常受欢迎的Java框架,其有很多优秀的特性让使用者更方便地进行应用的开发。其中,SpringBoot与CXF框架结合使用来调用Webservice可以非常简单地完成。本文将介绍SpringBoot与CXF框架结合使用来调用Webservice。
首先,我们需要在pom.xml文件中加入CXF及相关依赖。
```xml
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>3.2.14</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-transport-http</artifactId>
<version>3.2.14</version>
</dependency>
```
然后,我们需要在配置文件中设置一些参数,具体如下:
```yaml
cxf:
client:
simple.frontend: true
logging.enabled: true
timeout:
connect: 2000
receive: 5000
path: /ws
servlet:
url-pattern: /soap/*
```
在上述配置中,我们开启了日志记录,设置了连接超时和读取超时时间,以及指明了Webservice的路径。
接下来就可以创建一个接口来调用我们的Webservice。例如,我们想要调用一个返回学生列表的Webservice:
```java
@WebService
public interface StudentWebService {
@WebMethod
List<Student> getStudents();
}
```
我们使用CXF的JAX-WS Frontend创建了一个接口,同时使用@WebService注解来标记该接口。然后我们就可以创建一个实现类来实现该接口:
```java
@Service
public class StudentWebServiceImpl implements StudentWebService {
@Override
public List<Student> getStudents() {
// 调用Webservice,返回学生列表
return new ArrayList<>();
}
}
```
在上述实现类中,我们使用@Service注解来标记该类为SpringBoot的一个服务,同时实现了我们在接口中声明的getStudents()方法,去调用Webservice并返回学生列表。在该方法中,我们可以使用Spring提供的RestTemplate或者使用CXF的Client接口来进行调用。
然后我们同样使用CXF开放一个服务端口,供客户端调用:
```java
@Configuration
public class CxfConfig {
@Bean(name = Bus.DEFAULT_BUS_ID)
public SpringBus springBus() {
return new SpringBus();
}
@Bean
public ServletRegistrationBean<CXFServlet> cxfServlet() {
return new ServletRegistrationBean<CXFServlet>(new CXFServlet(), "/soap/*");
}
@Bean
public StudentWebService studentWebService() {
return new StudentWebServiceImpl();
}
@Bean
public Endpoint endpoint() {
EndpointImpl endpoint = new EndpointImpl(springBus(), studentWebService());
endpoint.publish("/StudentWebService");
return endpoint;
}
}
```
在上述代码中,我们创建了一个CXFServlet,并将其映射到/soap/*路径下,同时创建了一个WebService的实现类,在SpringBoot启动时通过Endpoint暴露出来。这样我们就可以在客户端中通过CXF框架来访问Webservice了。
总结一下,SpringBoot与CXF框架结合使用调用Webservice可以非常方便地完成。我们只需要提供一个接口,实现其方法并使用CXF暴露出去,就可以在客户端通过CXF框架来访问了。同时,我们还可以使用CXF的一些特性来定制化我们的调用过程,包括定制连接超时、读取超时、日志记录等。
阅读全文
相关推荐
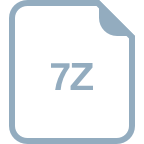

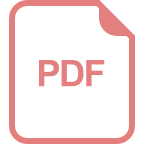
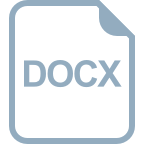
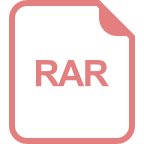
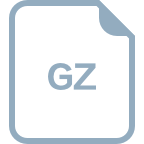
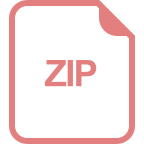




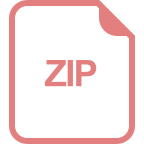
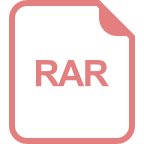
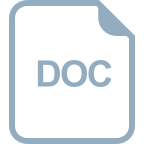
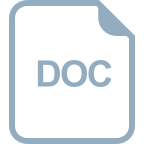