python的dlib库实现简单瘦脸功能
时间: 2023-07-12 14:08:41 浏览: 65
使用dlib库实现简单的瘦脸功能需要进行以下步骤:
1. 安装dlib库和其他相关库,如numpy和opencv。
2. 加载一张人脸照片并使用dlib的人脸检测器检测出人脸的关键点。
```python
import dlib
import cv2
import numpy as np
# 加载图片
img = cv2.imread("example.jpg")
# 初始化人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 检测人脸和关键点
faces = detector(img, 1)
for face in faces:
landmarks = predictor(img, face)
```
3. 根据关键点的位置计算出需要瘦脸的区域。
一般来说,瘦脸的方法是将下颚和脸颊的宽度减小一定比例,可以通过缩小下颚和脸颊关键点的横坐标实现。这里以缩小下颚为例:
```python
# 提取下颚关键点的坐标
jaw_points = []
for i in range(0, 17):
jaw_points.append((landmarks.part(i).x, landmarks.part(i).y))
# 计算下巴线的中心点
jaw_center = np.mean(jaw_points, axis=0)
# 将下颚关键点的横坐标向中心点移动
for i in range(0, 17):
x = landmarks.part(i).x
y = landmarks.part(i).y
landmarks.part(i).x = int(x - (x - jaw_center[0]) * 0.1)
```
4. 绘制并输出瘦脸后的结果。
```python
# 将结果绘制在原图上
for i in range(0, 68):
x = landmarks.part(i).x
y = landmarks.part(i).y
cv2.circle(img, (x, y), 1, (0, 0, 255), -1)
# 输出瘦脸后的结果
cv2.imwrite("result.jpg", img)
```
完整代码如下:
```python
import dlib
import cv2
import numpy as np
# 加载图片
img = cv2.imread("example.jpg")
# 初始化人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 检测人脸和关键点
faces = detector(img, 1)
for face in faces:
landmarks = predictor(img, face)
# 提取下颚关键点的坐标
jaw_points = []
for i in range(0, 17):
jaw_points.append((landmarks.part(i).x, landmarks.part(i).y))
# 计算下巴线的中心点
jaw_center = np.mean(jaw_points, axis=0)
# 将下颚关键点的横坐标向中心点移动
for i in range(0, 17):
x = landmarks.part(i).x
y = landmarks.part(i).y
landmarks.part(i).x = int(x - (x - jaw_center[0]) * 0.1)
# 将结果绘制在原图上
for i in range(0, 68):
x = landmarks.part(i).x
y = landmarks.part(i).y
cv2.circle(img, (x, y), 1, (0, 0, 255), -1)
# 输出瘦脸后的结果
cv2.imwrite("result.jpg", img)
```
相关推荐
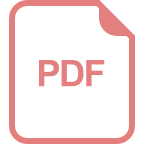
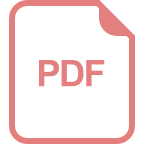














