已知dat1. dat存放了一系列整型数据。 要求: 1)用dat1. dat中的前100个数据给数组int a[100]赋值,并在屏幕上输出(每行10个元素)。 2)使用函数double isaver(int*p, int num) { } 求数组a中所有数组元素平均值。 3)把该函数放在头文件ISaver. h中以便在主函数中调用该函数。把所有小干平均值的数组元素(每行10个元素)和小于平均值的数组元素个数输出出来。(在屏幕上和数据文件d:\dat6. dat中同时输出)用c语言
时间: 2024-02-11 19:06:49 浏览: 72
以下是代码实现:
1. 从 dat1.dat 中读取前 100 个整型数据,存储到数组 a 中,并输出到屏幕上(每行 10 个元素):
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
int a[100];
int i = 0;
if ((fp = fopen("dat1.dat", "rb")) == NULL) {
printf("Failed to open file.\n");
return 1;
}
while (i < 100 && fread(&a[i], sizeof(int), 1, fp) == 1) {
printf("%d ", a[i]);
if ((i + 1) % 10 == 0) {
printf("\n");
}
i++;
}
fclose(fp);
return 0;
}
```
2. 编写函数 isaver,求出数组 a 中所有元素的平均值:
```c
double isaver(int *p, int num) {
int sum = 0;
int i = 0;
double aver;
for (i = 0; i < num; i++) {
sum += *(p + i);
}
aver = (double)sum / num;
return aver;
}
```
3. 将函数 isaver 放入头文件 ISaver.h 中,以便在主函数中调用:
ISaver.h 文件:
```c
#ifndef ISaver_h
#define ISaver_h
double isaver(int *p, int num);
#endif /* ISaver_h */
```
主函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include "ISaver.h"
int main() {
FILE *fp1, *fp2;
int a[100];
int i = 0;
double aver;
int count1 = 0, count2 = 0;
if ((fp1 = fopen("dat1.dat", "rb")) == NULL) {
printf("Failed to open file.\n");
return 1;
}
if ((fp2 = fopen("dat6.dat", "wb")) == NULL) {
printf("Failed to open file.\n");
return 1;
}
while (i < 100 && fread(&a[i], sizeof(int), 1, fp1) == 1) {
printf("%d ", a[i]);
if ((i + 1) % 10 == 0) {
printf("\n");
}
i++;
}
aver = isaver(a, 100);
printf("Average: %.2f\n", aver);
for (i = 0; i < 100; i++) {
if (a[i] < aver) {
count1++;
} else {
count2++;
}
}
printf("Elements smaller than average:\n");
for (i = 0; i < 100; i++) {
if (a[i] < aver) {
printf("%d ", a[i]);
if ((i + 1) % 10 == 0) {
printf("\n");
}
fwrite(&a[i], sizeof(int), 1, fp2);
}
}
printf("Number of elements smaller than average: %d\n", count1);
printf("Number of elements greater than or equal to average: %d\n", count2);
fclose(fp1);
fclose(fp2);
return 0;
}
```
阅读全文
相关推荐
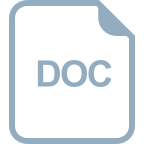
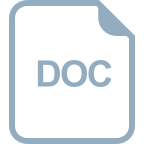
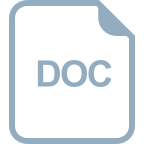






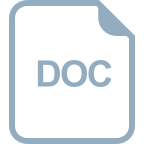
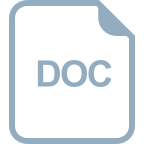
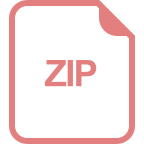
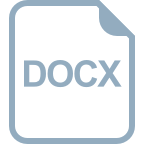
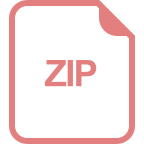
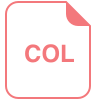
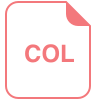
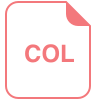
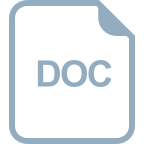
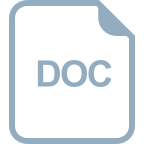