springboot集成shiro框架
时间: 2023-05-31 19:21:01 浏览: 167
### 回答1:
Spring Boot 集成 Shiro 框架可以实现在应用中实现安全认证、权限控制等功能。下面是大致的集成步骤:
1. 添加 Shiro 依赖
在 `pom.xml` 文件中添加 Shiro 的依赖。可以选择适合自己项目的版本号。
```xml
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-starter</artifactId>
<version>1.7.1</version>
</dependency>
```
2. 配置 Shiro
在 `application.properties` 或 `application.yml` 文件中添加 Shiro 的相关配置,如 Shiro 的过滤器、安全管理器、Realm 等。
```yaml
# 配置 Shiro 的过滤器
shiro:
filter:
anon: anon
authc: authc
# 配置 Shiro 的安全管理器和 Realm
shiro:
securityManager:
realm: myRealm
```
3. 实现 Realm
Realm 是 Shiro 的核心组件之一,需要实现自己的 Realm 类,用于提供认证和授权数据。
```java
public class MyRealm extends AuthorizingRealm {
// 认证
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
// 实现认证逻辑
}
// 授权
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) {
// 实现授权逻辑
}
}
```
4. 使用 Shiro
在需要进行安全认证、权限控制的地方,可以使用 Shiro 提供的 API 进行操作。
```java
// 获取当前用户的信息
Subject subject = SecurityUtils.getSubject();
Object principal = subject.getPrincipal();
// 判断当前用户是否具有某个角色
boolean hasRole = subject.hasRole("admin");
// 判断当前用户是否具有某个权限
boolean hasPermission = subject.isPermitted("user:update");
```
这是一个大致的 Spring Boot 集成 Shiro 的步骤,具体实现方式可以根据自己的需求进行调整。
### 回答2:
Spring Boot 是一个快速开发框架,Shiro 是一个安全框架,它们的集成可以帮助开发人员快速构建安全的 Web 应用程序。
为了将 Shiro 集成到 Spring Boot 中,首先需要在 Maven POM 文件中引入 Shiro 的依赖库。例如,以下 POM 文件中添加了 Shiro Spring Boot 的 Starter 依赖库:
```
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-starter</artifactId>
<version>1.5.0</version>
</dependency>
```
集成过程中,需要在应用程序中添加一个 Shiro 配置类,该类可以加载各种 Shiro 过滤器,用于对 URL 是否需要进行授权认证等操作,示例代码如下:
```java
@Configuration
public class ShiroConfig {
@Bean
public ShiroFilterFactoryBean shiroFilter(@Autowired SecurityManager securityManager){
ShiroFilterFactoryBean bean = new ShiroFilterFactoryBean();
bean.setSecurityManager(securityManager);
Map<String, String> chains = new HashMap<>();
chains.put("/login", "anon");
chains.put("/css/**", "anon");
chains.put("/js/**", "anon");
chains.put("/**", "authc");
bean.setFilterChainDefinitionMap(chains);
return bean;
}
@Bean
public SecurityManager securityManager(@Autowired UserRealm userRealm){
DefaultWebSecurityManager manager = new DefaultWebSecurityManager();
manager.setRealm(userRealm);
return manager;
}
@Bean
public UserRealm userRealm(){
return new UserRealm();
}
}
```
在这个例子中,我们为登录页、CSS、JS 等 URL 设置了“anon”过滤器,表示这些 URL 不需要进行认证,而其他 URL 都需要进行认证。此外,我们还提供了一个 UserRealm,用于提供用户的认证和授权。
最后,我们需要在应用程序中启用 Shiro,例如在启动主类里加入 @EnableShiro 注解:
```java
@EnableShiro
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
这样,我们就完成了 Shiro 的集成。使用 Shiro,开发人员可以方便地进行用户认证和授权,保障 Web 应用程序的安全性。
### 回答3:
Spring Boot是一个非常流行的Java Web框架,它可以通过添加各种插件来轻松集成许多其他框架和库。Shiro是一个全面的安全框架,提供了身份验证、授权、密码编码等功能,与Spring Boot的集成也非常容易。
下面是一些简要的步骤来集成Spring Boot和Shiro框架:
1. 首先,需要在pom.xml文件中添加Shiro和Spring Boot Starter Web的依赖:
```
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring</artifactId>
<version>1.4.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.3.2.RELEASE</version>
</dependency>
```
2. 然后,在应用程序的主类中添加@EnableWebSecurity注解,启用Spring Security的Web安全性支持,同时在configure(HttpSecurity http)方法中配置Shiro的安全过滤器链:
```
@Configuration
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.authorizeRequests()
.antMatchers("/login").permitAll()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/**").authenticated()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login").permitAll()
.defaultSuccessUrl("/home.html")
.and()
.logout()
.logoutUrl("/logout").permitAll()
.deleteCookies("JSESSIONID")
.logoutSuccessUrl("/login");
}
@Bean
public Realm realm() {
return new MyRealm();
}
@Bean
public DefaultWebSecurityManager securityManager() {
DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager();
securityManager.setRealm(realm());
return securityManager;
}
@Bean
public ShiroFilterFactoryBean shiroFilter() {
ShiroFilterFactoryBean shiroFilter = new ShiroFilterFactoryBean();
shiroFilter.setSecurityManager(securityManager());
shiroFilter.setLoginUrl("/login");
shiroFilter.setSuccessUrl("/home.html");
shiroFilter.setUnauthorizedUrl("/403.html");
Map<String, String> filterChainDefinitionMap = new LinkedHashMap<String, String>();
filterChainDefinitionMap.put("/login", "anon");
filterChainDefinitionMap.put("/logout", "logout");
filterChainDefinitionMap.put("/admin/**", "authc, roles[admin]");
filterChainDefinitionMap.put("/**", "user");
shiroFilter.setFilterChainDefinitionMap(filterChainDefinitionMap);
return shiroFilter;
}
}
```
3. 编写自定义的Realm类,用于实现Shiro的身份验证和授权逻辑:
```
public class MyRealm extends AuthorizingRealm {
@Autowired
private UserService userService;
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) {
SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo();
User user = (User) principals.getPrimaryPrincipal();
for (Role role : user.getRoles()) {
authorizationInfo.addRole(role.getName());
for (Permission permission : role.getPermissions()) {
authorizationInfo.addStringPermission(permission.getPermission());
}
}
return authorizationInfo;
}
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
UsernamePasswordToken upToken = (UsernamePasswordToken) token;
String username = upToken.getUsername();
User user = userService.findByUsername(username);
if (user != null) {
return new SimpleAuthenticationInfo(user, user.getPassword(), getName());
} else {
throw new UnknownAccountException();
}
}
}
```
4. 最后,在Controller中使用Shiro提供的Subject来实现身份验证和授权:
```
@Controller
public class HomeController {
@RequestMapping(value = "/home.html", method = RequestMethod.GET)
public String home() {
Subject currentUser = SecurityUtils.getSubject();
if (currentUser.isAuthenticated()) {
return "home";
} else {
return "redirect:/login";
}
}
@RequestMapping(value = "/admin/index.html", method = RequestMethod.GET)
public String admin() {
Subject currentUser = SecurityUtils.getSubject();
if (currentUser.isAuthenticated() && currentUser.hasRole("admin")) {
return "admin";
} else {
return "redirect:/login";
}
}
@RequestMapping(value = "/login", method = RequestMethod.GET)
public String login() {
return "login";
}
}
```
使用以上步骤实现了Spring Boot集成Shiro框架,完成了基本的身份验证和授权操作。实现后就可以进行测试,通过Shiro的身份验证和授权功能保证系统的安全性。
阅读全文
相关推荐
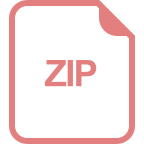
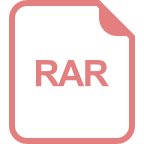
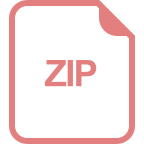
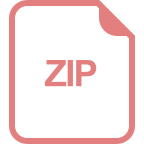
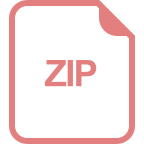
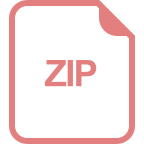
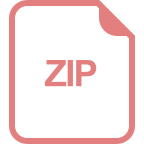
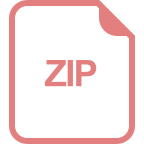
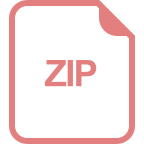
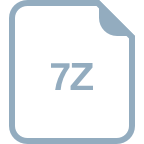
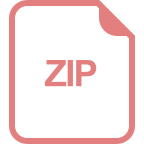
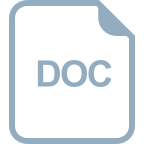
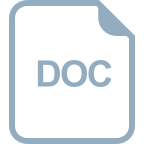
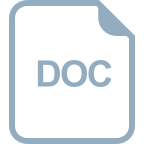
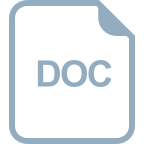

