.net 实现TCP通讯中:客户端软件获取本地ip 并 指定本地端口号,实时接收服务端的数据
时间: 2024-03-10 21:44:55 浏览: 220
在 .NET 中实现TCP通讯,可以使用 TcpClient 类来作为客户端进行连接和通讯。
要获取本地IP地址,可以使用 System.Net.NetworkInformation 命名空间中的 NetworkInterface 类,通过遍历网卡获取到本地IP地址。
指定本地端口号可以在实例化 TcpClient 对象时传入一个本地端口号。
下面是一个示例代码:
```csharp
using System;
using System.Net;
using System.Net.NetworkInformation;
using System.Net.Sockets;
class Program
{
static void Main(string[] args)
{
// 获取本地IP地址
string localIp = "";
NetworkInterface[] interfaces = NetworkInterface.GetAllNetworkInterfaces();
foreach (NetworkInterface iface in interfaces)
{
if (iface.NetworkInterfaceType == NetworkInterfaceType.Wireless80211 || iface.NetworkInterfaceType == NetworkInterfaceType.Ethernet)
{
foreach (UnicastIPAddressInformation ip in iface.GetIPProperties().UnicastAddresses)
{
if (ip.Address.AddressFamily == AddressFamily.InterNetwork)
{
localIp = ip.Address.ToString();
break;
}
}
}
}
Console.WriteLine("本地IP地址:" + localIp);
// 指定本地端口号并连接服务器
TcpClient client = new TcpClient(localIp, 8888);
Console.WriteLine("连接服务器成功");
// 接收服务器数据
NetworkStream stream = client.GetStream();
byte[] buffer = new byte[1024];
int len = stream.Read(buffer, 0, buffer.Length);
string data = System.Text.Encoding.Default.GetString(buffer, 0, len);
Console.WriteLine("接收到的数据:" + data);
// 关闭连接
stream.Close();
client.Close();
}
}
```
在上面的代码中,我们先获取了本地IP地址,然后实例化 TcpClient 对象时传入了本地IP地址和端口号,连接了服务器。接下来通过 TcpClient 的 GetStream 方法获取到了网络流,并通过 Read 方法读取了服务器发送的数据。最后关闭了网络流和 TcpClient 对象。
阅读全文
相关推荐
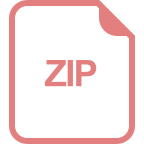

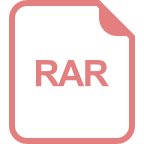
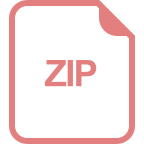
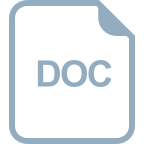
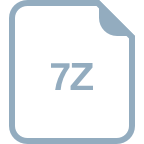
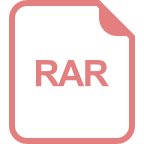
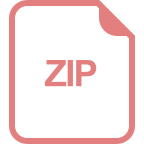
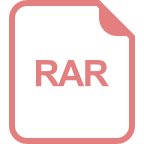
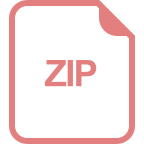
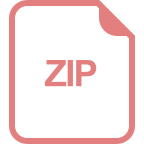
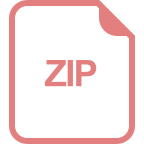
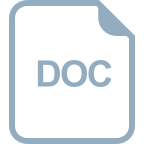
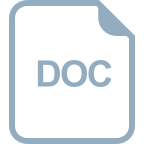
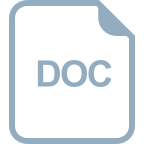
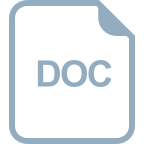
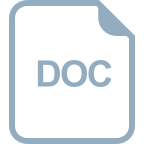