基于python人脸识别的实验目的
时间: 2023-11-01 10:08:16 浏览: 80
基于Python人脸识别的实验目的主要有以下几个方面:
1. 掌握人脸识别技术的基本原理和方法,了解人脸识别技术的发展历程和应用现状;
2. 熟悉Python编程语言及其相关的图像处理库、深度学习框架等工具,了解如何利用这些工具实现人脸识别算法;
3. 实践人脸识别算法的应用,例如人脸识别门禁系统、人脸识别支付系统、人脸表情识别等,加深对人脸识别技术的理解和应用;
4. 提升对计算机视觉及人工智能的兴趣和认识,为进一步深入学习相关领域打下基础。
相关问题
基于python人脸识别的实验意义
Python人脸识别的实验意义在于探索利用计算机视觉技术进行人脸识别的方法和应用。通过实验,我们可以学习和掌握人脸识别的基本原理、算法、技术和工具,了解人脸识别在安防、人机交互、生物识别等领域的应用,提高计算机视觉和机器学习的能力和水平。此外,基于Python的人脸识别实验也可以帮助我们了解Python编程语言和图像处理库的使用方法,提高编程能力和实践经验。
基于python人脸识别
基于Python的人脸识别技术主要包括三个部分:人脸检测、人脸特征提取和人脸识别。常用的库包括OpenCV和Dlib等。
以下是一个基于Python的人脸识别的示例代码:
```python
import cv2
import dlib
# 加载正脸检测器
detector = dlib.get_frontal_face_detector()
# 加载人脸关键点检测器
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载人脸识别模型
facerec = dlib.face_recognition_model_v1("dlib_face_recognition_resnet_model_v1.dat")
# 加载图片
img = cv2.imread("test.jpg")
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray, 1)
# 遍历每个人脸
for face in faces:
# 获取人脸关键点
landmarks = predictor(gray, face)
# 获取人脸特征
face_descriptor = facerec.compute_face_descriptor(img, landmarks)
# 在图像中绘制人脸框和关键点
cv2.rectangle(img, (face.left(), face.top()), (face.right(), face.bottom()), (0, 255, 0), 2)
for i in range(68):
x = landmarks.part(i).x
y = landmarks.part(i).y
cv2.circle(img, (x, y), 2, (0, 0, 255), -1)
# 显示图像
cv2.imshow("image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该代码使用了Dlib库进行人脸检测、关键点检测和人脸识别,并使用OpenCV库进行图像处理和显示。具体步骤包括:
1. 加载正脸检测器、人脸关键点检测器和人脸识别模型。
2. 加载图片并转换为灰度图像。
3. 使用正脸检测器检测人脸。
4. 遍历每个人脸,获取人脸关键点和人脸特征,并在图像中绘制人脸框和关键点。
5. 显示图像。
相关推荐
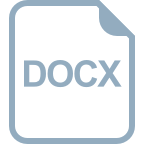
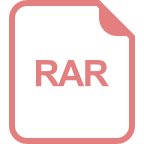

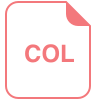
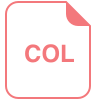
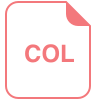
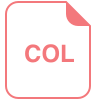






