定义一个Rectangle类,长方形类有四个点对象作为属性,编写方法实现以下功能: 用c++写出判断四个点能不能成为一个长方形的四个顶点,计算出长方形的长,计算出长方形的宽,计算长方形的面积,计算长方形的周长
时间: 2023-05-14 19:05:11 浏览: 216
以下是一个可能的 Rectangle 类的定义和实现:
```c
#include <stdio.h>
#include <math.h>
typedef struct Point {
double x;
double y;
} Point;
typedef struct Rectangle {
Point p1;
Point p2;
Point p3;
Point p4;
} Rectangle;
int is_rectangle(Rectangle r) {
double d1 = sqrt(pow(r.p1.x - r.p2.x, 2) + pow(r.p1.y - r.p2.y, 2));
double d2 = sqrt(pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2));
double d3 = sqrt(pow(r.p3.x - r.p4.x, 2) + pow(r.p3.y - r.p4.y, 2));
double d4 = sqrt(pow(r.p4.x - r.p1.x, 2) + pow(r.p4.y - r.p1.y, 2));
double d5 = sqrt(pow(r.p1.x - r.p3.x, 2) + pow(r.p1.y - r.p3.y, 2));
double d6 = sqrt(pow(r.p2.x - r.p4.x, 2) + pow(r.p2.y - r.p4.y, 2));
return (d1 == d3 && d2 == d4 && d5 == d6);
}
double rectangle_length(Rectangle r) {
double d1 = sqrt(pow(r.p1.x - r.p2.x, 2) + pow(r.p1.y - r.p2.y, 2));
double d2 = sqrt(pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2));
return (d1 == d2) ? d1 : d2;
}
double rectangle_width(Rectangle r) {
double d1 = sqrt(pow(r.p1.x - r.p2.x, 2) + pow(r.p1.y - r.p2.y, 2));
double d2 = sqrt(pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2));
return (d1 == d2) ? d2 : d1;
}
double rectangle_area(Rectangle r) {
return rectangle_length(r) * rectangle_width(r);
}
double rectangle_perimeter(Rectangle r) {
return 2 * (rectangle_length(r) + rectangle_width(r));
}
int main() {
Rectangle r = {{0, 0}, {0, 1}, {1, 1}, {1, 0}};
if (is_rectangle(r)) {
printf("Length: %f\n", rectangle_length(r));
printf("Width: %f\n", rectangle_width(r));
printf("Area: %f\n", rectangle_area(r));
printf("Perimeter: %f\n", rectangle_perimeter(r));
} else {
printf("Not a rectangle!\n");
}
return 0;
}
```
这个程序定义了一个 Point 结构体和一个 Rectangle 结构体,其中 Rectangle 结构体包含了四个 Point 对象作为属性。is_rectangle 函数用于判断四个点能否组成一个长方形,它通过计算每条边的长度来判断是否相等。rectangle_length 函数和 rectangle_width 函数分别计算长方形的长和宽,它们通过比较两条边的长度来确定哪条是长边,哪条是短边。rectangle_area 函数和 rectangle_perimeter 函数分别计算长方形的面积和周长,它们使用了 rectangle_length 函数和 rectangle_width 函数来计算长和宽。
在 main 函数中,我们定义了一个 Rectangle 对象 r,然后调用 is_rectangle 函数来判断它是否是一个长方形。如果是,我们就调用 rectangle_length 函数、rectangle_width 函数、rectangle_area 函数和 rectangle_perimeter 函数来计算长、宽、面积和周长,并输出结果。如果不是,我们就输出一个错误信息。
阅读全文
相关推荐
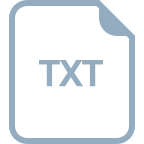
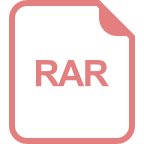
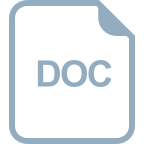















