C语言实现从文件中读取多类型数据并存储到数组中
时间: 2024-05-13 10:17:03 浏览: 8
可以使用文件操作函数 `fopen` 和 `fscanf` 将文件中的数据读入数组中。例如,如果文件中的数据类型包括整型和浮点型,可以按照以下步骤进行操作:
1. 打开文件并检查文件是否成功打开:
```
FILE *fp;
fp = fopen("filename", "r"); // 打开文件
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
```
2. 定义数组并读取文件中的数据:
```
int int_array[MAX_SIZE];
float float_array[MAX_SIZE];
int i = 0;
while (fscanf(fp, "%d%f", &int_array[i], &float_array[i]) == 2) {
i++;
}
```
3. 关闭文件:
```
fclose(fp);
```
完整代码示例:
```
#include <stdio.h>
#define MAX_SIZE 100
int main() {
FILE *fp;
fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
int int_array[MAX_SIZE];
float float_array[MAX_SIZE];
int i = 0;
while (fscanf(fp, "%d%f", &int_array[i], &float_array[i]) == 2) {
i++;
}
fclose(fp);
// 输出数组中的数据
for (int j = 0; j < i; j++) {
printf("%d %.2f\n", int_array[j], float_array[j]);
}
return 0;
}
```
注意:在读取文件中的数据时,需要根据文件中数据的实际类型来确定 `fscanf` 函数中的格式字符串。例如,"%d" 表示读取整型数据,"%.2f" 表示读取保留两位小数的浮点型数据。
相关推荐
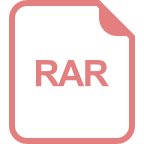
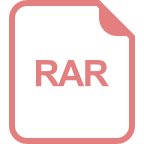














