opencv for unity 人的轮廓检测
时间: 2023-10-01 22:12:29 浏览: 222
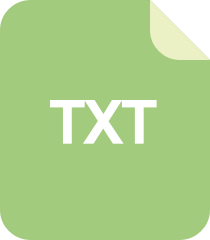
OpenCV for Unity 2.3.3.unitypackage
要在Unity中检测人的轮廓,可以使用OpenCV for Unity插件。以下是一些步骤:
1. 下载和安装OpenCV for Unity插件(https://assetstore.unity.com/packages/tools/integration/opencv-for-unity-21088)。
2. 将图像作为纹理加载到Unity中。
3. 使用OpenCV for Unity插件中的"TextureToMat"功能将纹理转换为OpenCV Mat对象。
4. 使用OpenCV for Unity中的人脸检测功能检测人脸。
5. 使用OpenCV for Unity中的轮廓检测功能检测人的轮廓。
6. 将检测到的轮廓绘制到纹理上。
7. 将纹理显示在Unity场景中。
下面是一个代码示例,展示如何使用OpenCV for Unity插件进行人的轮廓检测:
```
using UnityEngine;
using System.Collections;
using OpenCVForUnity.CoreModule;
using OpenCVForUnity.ImgprocModule;
using OpenCVForUnity.UnityUtils;
public class ContourDetectionExample : MonoBehaviour
{
// Use this for initialization
void Start()
{
// Load the texture into a Texture2D object
Texture2D texture = Resources.Load("test") as Texture2D;
// Create a new Mat object from the texture
Mat rgbaMat = new Mat(texture.height, texture.width, CvType.CV_8UC4);
// Convert the texture to a Mat object
Utils.texture2DToMat(texture, rgbaMat);
// Convert the Mat object to grayscale
Mat grayMat = new Mat();
Imgproc.cvtColor(rgbaMat, grayMat, Imgproc.COLOR_RGBA2GRAY);
// Threshold the image to create a binary image
Mat binaryMat = new Mat();
Imgproc.threshold(grayMat, binaryMat, 0, 255, Imgproc.THRESH_BINARY);
// Find the contours in the binary image
List<MatOfPoint> contours = new List<MatOfPoint>();
Mat hierarchy = new Mat();
Imgproc.findContours(binaryMat, contours, hierarchy, Imgproc.RETR_EXTERNAL, Imgproc.CHAIN_APPROX_SIMPLE);
// Draw the contours on the original image
Imgproc.drawContours(rgbaMat, contours, -1, new Scalar(0, 255, 0, 255), 2);
// Convert the Mat object back to a Texture2D object
Texture2D outputTexture = new Texture2D(rgbaMat.cols(), rgbaMat.rows(), TextureFormat.RGBA32, false);
Utils.matToTexture2D(rgbaMat, outputTexture);
// Display the output texture in the scene
GetComponent<Renderer>().material.mainTexture = outputTexture;
}
}
```
这个代码示例加载了一个名为“test”的纹理,将其转换为Mat对象,然后使用OpenCV for Unity中的轮廓检测功能检测人的轮廓,并在原始图像上绘制轮廓。最后,将Mat对象转换回Texture2D对象并在场景中显示它。
阅读全文
相关推荐
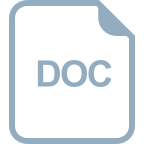
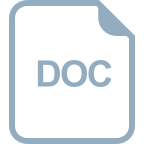
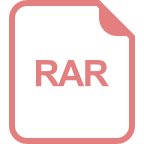
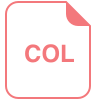
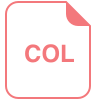
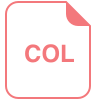
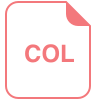
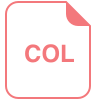
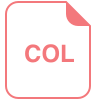
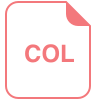
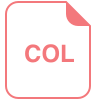
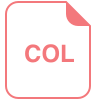
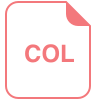
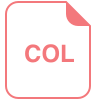
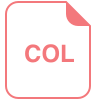

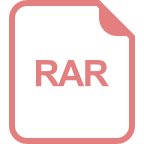