websocketpp::lib::bind()
时间: 2023-09-14 08:07:41 浏览: 112
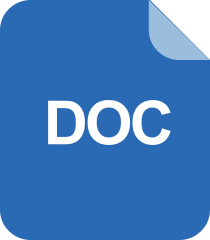
网络编程socket之bind函数
websocketpp::lib::bind() is a function that binds a socket to a specific IP address and port number. It is used in network programming to establish a connection between a client and a server. The bind() function takes three arguments: the socket file descriptor, a pointer to a sockaddr structure that contains the IP address and port number to bind to, and the size of the sockaddr structure.
Here's an example of how to use bind() in C++:
```cpp
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
std::cerr << "Error creating socket\n";
return 1;
}
sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = inet_addr("127.0.0.1"); // bind to localhost
serv_addr.sin_port = htons(8080); // bind to port 8080
int bind_result = bind(sockfd, (struct sockaddr *) &serv_addr, sizeof(serv_addr));
if (bind_result < 0) {
std::cerr << "Error binding socket\n";
return 1;
}
std::cout << "Socket bound to port 8080\n";
return 0;
}
```
In this example, we create a socket using the socket() function and specify the address family (IPv4), socket type (TCP), and protocol (0 for default). We then create a sockaddr_in structure and specify the IP address and port number to bind to. Finally, we call bind() with the socket file descriptor, a pointer to the sockaddr_in structure, and the size of the structure. If the bind() function returns a negative value, it indicates an error. Otherwise, the socket is successfully bound to the specified IP address and port number.
阅读全文
相关推荐
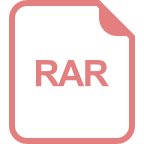
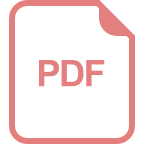



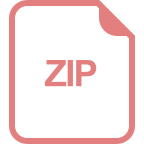
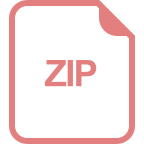
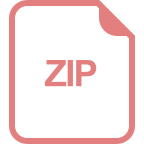
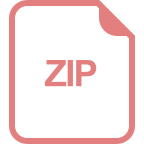
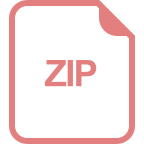
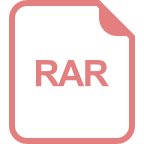
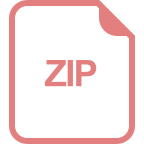
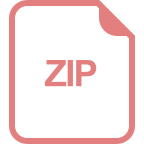
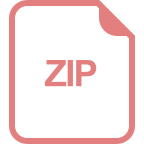
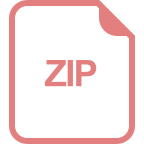
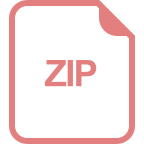
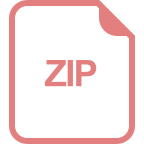
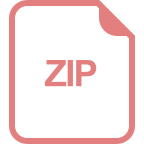