编写一个程序,使用字节流实现拷贝一个文件(文本文件,文件内容中有中英文字符),并输出拷贝花费的时间 提示: 1)使用FilelnputStream、FileOutputStream。 2)贝时,分别使用每次读取按字节及字节数组缓冲区方法,比较两种方法下,花费时间的大小。
时间: 2023-07-16 21:17:12 浏览: 147
以下是Java代码实现:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String srcFilePath = "source.txt"; // 源文件路径
String destFilePath = "destination.txt"; // 目标文件路径
// 测试使用每次读取一个字节的方法
long startTime1 = System.currentTimeMillis(); // 记录开始时间
copyByByte(srcFilePath, destFilePath);
long endTime1 = System.currentTimeMillis(); // 记录结束时间
System.out.println("每次读取一个字节花费时间:" + (endTime1 - startTime1) + "ms");
// 测试使用字节数组缓冲区方法
long startTime2 = System.currentTimeMillis(); // 记录开始时间
copyByBuffer(srcFilePath, destFilePath);
long endTime2 = System.currentTimeMillis(); // 记录结束时间
System.out.println("使用字节数组缓冲区方法花费时间:" + (endTime2 - startTime2) + "ms");
}
// 每次读取一个字节的方法
public static void copyByByte(String srcFilePath, String destFilePath) {
try (FileInputStream fis = new FileInputStream(srcFilePath);
FileOutputStream fos = new FileOutputStream(destFilePath)) {
int b;
while ((b = fis.read()) != -1) {
fos.write(b);
}
} catch (IOException e) {
e.printStackTrace();
}
}
// 使用字节数组缓冲区方法
public static void copyByBuffer(String srcFilePath, String destFilePath) {
try (FileInputStream fis = new FileInputStream(srcFilePath);
FileOutputStream fos = new FileOutputStream(destFilePath)) {
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先定义了源文件路径和目标文件路径。然后,我们分别测试了使用每次读取一个字节和使用字节数组缓冲区方法拷贝文件的时间。在每个方法中,我们都使用了Java 7的try-with-resources语句来确保文件流的正确关闭。
当我们运行这个程序时,它会输出每个方法花费的时间。可以看到,使用字节数组缓冲区方法比每次读取一个字节的方法快得多。这是因为使用缓冲区可以减少文件I/O操作的次数,从而提高效率。
阅读全文
相关推荐
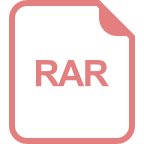
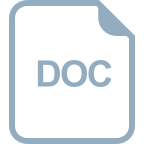
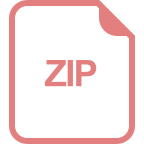
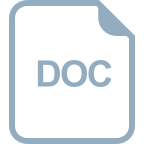
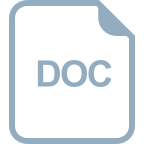
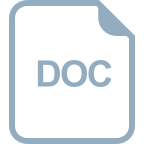
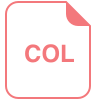
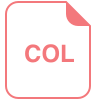
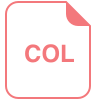
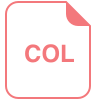
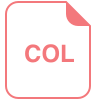