怎么用java代码获取zip文件头信息
时间: 2023-11-21 09:05:07 浏览: 42
在Java中可以使用ZipFile类获取ZIP文件的头信息,具体步骤如下:
1. 创建ZipFile对象,需要传入ZIP文件的路径。
```
ZipFile zipFile = new ZipFile("your_zip_file_path");
```
2. 获取ZipEntry对象,可以通过ZipFile对象的getEntry方法获取。
```
ZipEntry zipEntry = zipFile.getEntry("your_zip_entry_name");
```
3. 获取ZipEntry对象的头信息,可以通过ZipEntry对象的一些方法获取,如getCompressionMethod、getCrc、getCompressedSize、getSize等。
```
int compressionMethod = zipEntry.getCompressionMethod();
long crc = zipEntry.getCrc();
long compressedSize = zipEntry.getCompressedSize();
long size = zipEntry.getSize();
```
完整的代码示例如下:
```java
import java.io.IOException;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
public class ZipHeaderInfo {
public static void main(String[] args) {
String zipFilePath = "your_zip_file_path";
try (ZipFile zipFile = new ZipFile(zipFilePath)) {
Enumeration<? extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
System.out.println("Entry name: " + entry.getName());
System.out.println("Compression method: " + entry.getCompressionMethod());
System.out.println("CRC: " + entry.getCrc());
System.out.println("Compressed size: " + entry.getCompressedSize());
System.out.println("Size: " + entry.getSize());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该代码可以输出ZIP文件中每个条目的头信息,可以根据需要修改代码以获取特定条目的头信息。
相关推荐
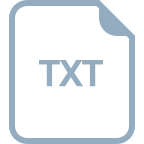
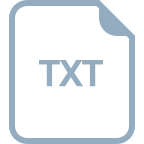














