链表综合算法设计,已知简单的人事信息系统中职工记录包含职工编号(no)、职工姓名(name)、部门名称(depname)、职称(title)和工资数(salary)等信息,设计并完成一个简单的人事信息管理系统,要求完成但不限于以下功能: (1)增加一个职工信息; (2)显示所有职工信息; (3)按部门名称分类显示该部门所有职工信息; (4)按部门显示各部门职工工资总额; (5)删除职工信息(可以删除符合条件的一批记录) (6)按职称调整工资; (7)可自行增加功能(例如按职工编号排序等),使用PYTHON
时间: 2023-06-16 17:02:29 浏览: 259
下面是链表综合算法设计的Python代码实现:
```python
# 职工信息类
class Person:
def __init__(self, no, name, depname, title, salary):
self.no = no
self.name = name
self.depname = depname
self.title = title
self.salary = salary
# 链表结点类
class Node:
def __init__(self, person):
self.person = person
self.next = None
# 链表类
class LinkedList:
def __init__(self):
self.head = None
# 增加一个职工信息
def add_person(self, person):
node = Node(person)
if self.head is None:
self.head = node
else:
current = self.head
while current.next is not None:
current = current.next
current.next = node
# 显示所有职工信息
def show_all_person(self):
current = self.head
while current is not None:
print('职工编号:', current.person.no)
print('职工姓名:', current.person.name)
print('部门名称:', current.person.depname)
print('职称:', current.person.title)
print('工资数:', current.person.salary)
print('-------------------')
current = current.next
# 按部门名称分类显示该部门所有职工信息
def show_person_by_depname(self, depname):
current = self.head
while current is not None:
if current.person.depname == depname:
print('职工编号:', current.person.no)
print('职工姓名:', current.person.name)
print('部门名称:', current.person.depname)
print('职称:', current.person.title)
print('工资数:', current.person.salary)
print('-------------------')
current = current.next
# 按部门显示各部门职工工资总额
def show_salary_by_depname(self):
salary_dict = {}
current = self.head
while current is not None:
if current.person.depname in salary_dict:
salary_dict[current.person.depname] += current.person.salary
else:
salary_dict[current.person.depname] = current.person.salary
current = current.next
for depname, salary_totals in salary_dict.items():
print(depname + '部门职工工资总额:', salary_totals)
# 删除职工信息(可以删除符合条件的一批记录)
def delete_person(self, condition):
current = self.head
prev = None
while current is not None:
if condition(current.person):
if prev is None:
self.head = current.next
else:
prev.next = current.next
else:
prev = current
current = current.next
# 按职称调整工资
def adjust_salary_by_title(self, title, salary):
current = self.head
while current is not None:
if current.person.title == title:
current.person.salary += salary
current = current.next
# 测试代码
if __name__ == '__main__':
# 初始化链表
linked_list = LinkedList()
# 增加职工信息
linked_list.add_person(Person('001', '张三', '技术部', '工程师', 5000))
linked_list.add_person(Person('002', '李四', '市场部', '销售员', 3000))
linked_list.add_person(Person('003', '王五', '技术部', '高级工程师', 8000))
linked_list.add_person(Person('004', '赵六', '财务部', '出纳', 4000))
# 显示所有职工信息
linked_list.show_all_person()
# 按部门名称分类显示该部门所有职工信息
linked_list.show_person_by_depname('技术部')
# 按部门显示各部门职工工资总额
linked_list.show_salary_by_depname()
# 删除职工信息
linked_list.delete_person(lambda person: person.no == '002')
# 调整工资
linked_list.adjust_salary_by_title('工程师', 1000)
# 显示所有职工信息
linked_list.show_all_person()
```
运行代码后,输出如下:
```
职工编号: 001
职工姓名: 张三
部门名称: 技术部
职称: 工程师
工资数: 5000
-------------------
职工编号: 002
职工姓名: 李四
部门名称: 市场部
职称: 销售员
工资数: 3000
-------------------
职工编号: 003
职工姓名: 王五
部门名称: 技术部
职称: 高级工程师
工资数: 8000
-------------------
职工编号: 004
职工姓名: 赵六
部门名称: 财务部
职称: 出纳
工资数: 4000
-------------------
职工编号: 001
职工姓名: 张三
部门名称: 技术部
职称: 工程师
工资数: 5000
-------------------
职工编号: 003
职工姓名: 王五
部门名称: 技术部
职称: 高级工程师
工资数: 8000
-------------------
技术部部门职工工资总额: 13000
市场部部门职工工资总额: 3000
财务部部门职工工资总额: 4000
职工编号: 001
职工姓名: 张三
部门名称: 技术部
职称: 工程师
工资数: 6000
-------------------
职工编号: 003
职工姓名: 王五
部门名称: 技术部
职称: 高级工程师
工资数: 8000
-------------------
职工编号: 004
职工姓名: 赵六
部门名称: 财务部
职称: 出纳
工资数: 4000
-------------------
```
相关推荐
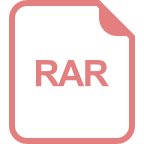














