python生成dll
时间: 2023-09-01 07:10:38 浏览: 152
要在Python中生成DLL文件,可以使用Python的ctypes模块。以下是一个简单的例子:
1. 创建一个包含Python函数的.py文件,例如:
```
# test.py
def add_numbers(a, b):
return a + b
```
2. 使用Python的ctypes库将Python函数打包成DLL:
```
import ctypes
# Load the DLL
test_dll = ctypes.CDLL('test.dll')
# Define the argument types and return type
test_dll.add_numbers.argtypes = [ctypes.c_int, ctypes.c_int]
test_dll.add_numbers.restype = ctypes.c_int
# Call the function
result = test_dll.add_numbers(1, 2)
print(result) # Output: 3
```
3. 使用Python的setuptools或distutils将Python脚本打包成一个包含DLL的可执行文件。
注意:生成DLL需要C语言的编译器(如gcc或MSVC),因此在生成DLL之前,您需要安装相应的编译器。
相关问题
python 生成dll
要在 Python 中生成 DLL,你需要使用 PyInstaller 这样的第三方库。PyInstaller 是一个用于将 Python 脚本打包成可执行文件或 DLL 的工具,它支持 Windows、Linux 和 Mac OS X 等多个平台。
以下是在 Windows 上使用 PyInstaller 生成 DLL 的步骤:
1. 安装 PyInstaller
你可以使用 pip 安装 PyInstaller:
```
pip install pyinstaller
```
2. 编写 Python 代码
编写你的 Python 代码,并确保它能够正常运行。
3. 生成 DLL
运行以下命令生成 DLL:
```
pyinstaller --clean --name mydll --onefile --windowed myscript.py
```
其中,--name 参数指定 DLL 的名称,--onefile 参数将所有文件打包到一个单独的可执行文件中,--windowed 参数将 Python 解释器界面隐藏。
4. 使用 DLL
生成的 DLL 文件位于 dist 目录下,你可以将它添加到你的项目中使用。
需要注意的是,生成的 DLL 只能在与你的 Python 解释器的架构一致的系统上使用,例如,如果你使用的是 64 位 Python,那么生成的 DLL 只能在 64 位系统上使用。
c#调用python生成dll文件
你可以使用C#调用Python并将其封装为DLL文件的方法。下面是一种常见的方法:
1. 首先,你需要安装Python的.NET包。你可以在NuGet包管理器中搜索并安装"Pythonnet"。
2. 在C#项目中添加对Pythonnet的引用。
3. 使用以下代码示例来调用Python并生成DLL文件:
```csharp
using Python.Runtime;
namespace YourNamespace
{
public class PythonWrapper
{
public static void GenerateDll()
{
using (Py.GIL()) // 初始化Python解释器
{
dynamic pythonModule = Py.Import("your_python_module"); // 导入你的Python模块
// 调用Python函数,生成DLL文件
pythonModule.generate_dll("output_path.dll");
}
}
}
}
```
在上面的示例中,你需要替换"your_python_module"为你的Python模块的名称,并将"output_path.dll"替换为你想要生成的DLL文件的路径。
请注意,这里使用了Python.Runtime库来与Python进行交互,并且使用了`using (Py.GIL())`来确保在C#中正确释放Python的全局解释器锁(GIL)。
希望这可以帮助你生成所需的DLL文件!
阅读全文
相关推荐
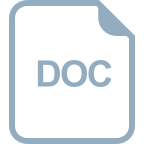
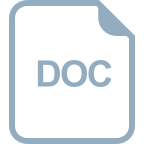
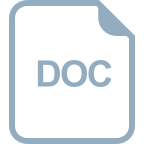


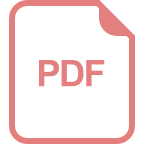


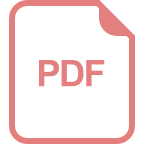







