点云机器学习提取窨井盖代码
时间: 2023-11-12 11:13:09 浏览: 133
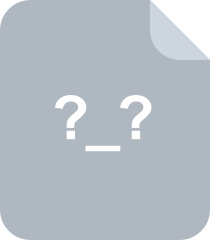
Las点云数据读取代码

点云机器学习提取窨井盖的代码,可以分为以下几个步骤:
1. 点云数据读取:使用开源的点云处理库PCL(Point Cloud Library)读取点云数据。
```cpp
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ>("input_cloud.pcd", *cloud) == -1)
{
PCL_ERROR("Could not read file\n");
return -1;
}
```
2. 点云滤波:使用PCL库中的滤波器对采集的点云进行处理,去除噪点和离群点。
```cpp
#include <pcl/filters/voxel_grid.h>
#include <pcl/filters/passthrough.h>
#include <pcl/filters/statistical_outlier_removal.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered(new pcl::PointCloud<pcl::PointXYZ>);
// Voxel Grid滤波器
pcl::VoxelGrid<pcl::PointXYZ> sor;
sor.setInputCloud(cloud);
sor.setLeafSize(0.1f, 0.1f, 0.1f);
sor.filter(*cloud_filtered);
// PassThrough滤波器
pcl::PassThrough<pcl::PointXYZ> pass;
pass.setInputCloud(cloud_filtered);
pass.setFilterFieldName("z");
pass.setFilterLimits(0.0, 2.0);
pass.filter(*cloud_filtered);
// Statistical Outlier Removal滤波器
pcl::StatisticalOutlierRemoval<pcl::PointXYZ> sor;
sor.setInputCloud(cloud_filtered);
sor.setMeanK(50);
sor.setStddevMulThresh(1.0);
sor.filter(*cloud_filtered);
```
3. 特征提取:使用PCL库中的特征提取器对点云进行处理,提取出窨井盖的特征点。
```cpp
#include <pcl/features/normal_3d.h>
#include <pcl/features/fpfh.h>
// 计算法向量
pcl::PointCloud<pcl::Normal>::Ptr normals(new pcl::PointCloud<pcl::Normal>);
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>);
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud(cloud_filtered);
ne.setSearchMethod(tree);
ne.setRadiusSearch(0.1);
ne.compute(*normals);
// 计算FPFH特征
pcl::PointCloud<pcl::FPFHSignature33>::Ptr fpfhs(new pcl::PointCloud<pcl::FPFHSignature33>);
pcl::FPFHEstimation<pcl::PointXYZ, pcl::Normal, pcl::FPFHSignature33> fpfh;
fpfh.setInputCloud(cloud_filtered);
fpfh.setInputNormals(normals);
fpfh.setSearchMethod(tree);
fpfh.setRadiusSearch(0.2);
fpfh.compute(*fpfhs);
// 使用一些聚类算法对特征点进行聚类,得到窨井盖的位置
```
4. 输出结果:将提取出来的窨井盖的位置输出到文件中,以便后续使用。
```cpp
std::ofstream ofs("output.txt");
for (size_t i = 0; i < indices.size(); ++i)
{
int idx = indices[i];
ofs << cloud_filtered->points[idx].x << " "
<< cloud_filtered->points[idx].y << " "
<< cloud_filtered->points[idx].z << std::endl;
}
ofs.close();
```
需要注意的是,以上代码仅为示例,具体实现可能会有所不同,需要根据实际情况进行调整。同时,对于窨井盖的特征提取也可以使用其他的算法,例如基于深度学习的目标检测算法。
阅读全文
相关推荐
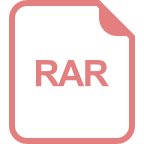
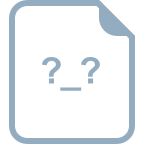
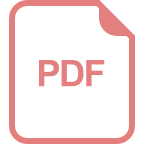
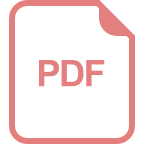
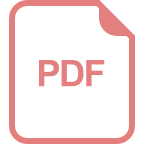
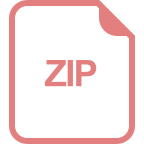
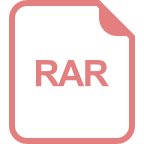
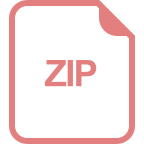
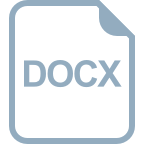
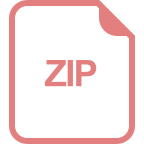
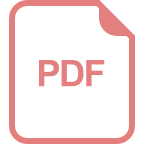
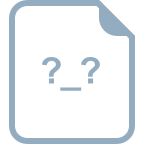
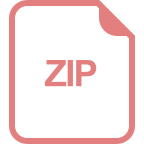
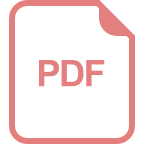