爬虫技术抓取https://www.secoo.com/网站数据
时间: 2024-09-28 17:06:19 浏览: 54
爬虫技术是一种用于从互联网上自动获取信息的程序,通常通过发送HTTP请求到网页服务器并解析返回的数据(HTML、XML或JSON等)。对于抓取https://www.secoo.com/网站的数据,一般步骤包括:
1. **设置请求库**:使用Python语言,你可以使用requests库发起HTTPS请求。例如:
```python
import requests
url = 'https://www.secoo.com/'
response = requests.get(url)
```
2. **处理响应**:检查响应状态码(如200表示成功),然后解析内容,常见的解析工具有BeautifulSoup或lxml库对HTML的解析,pandas库对表格数据的处理。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
```
3. **提取所需数据**:定位目标数据所在的HTML元素,通过CSS选择器或XPath表达式找到,并将其存储起来。
```python
data_elements = soup.select('target-element-selector')
data = [element.text for element in data_elements]
```
4. **错误处理**:考虑到网络不稳定和网站反爬机制,需要处理可能出现的异常,比如重试、登录验证或rate limiting。
相关问题
编写爬虫程序,提取“https://www.secoo.com/”网站首页的数据与资源
编写一个Python爬虫提取“https://www.secoo.com/”网站首页数据和资源,首先需要了解一些基本步骤:
1. **安装依赖**:安装requests用于发送HTTP请求,BeautifulSoup或lxml用于解析HTML。
```bash
pip install requests beautifulsoup4
```
2. **发送请求**:
使用`requests.get()`获取网页源代码。
```python
import requests
url = "https://www.secoo.com/"
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print("请求失败")
```
3. **解析HTML**:
通过BeautifulSoup解析HTML内容,并提取所需信息。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
# 提取页面标题、链接等元素
title = soup.title.string
links = [a['href'] for a in soup.find_all('a', href=True)]
images = [img['src'] for img in soup.find_all('img', src=True)]
```
4. **提取资源**:
对于图片和其他资源,你可能需要进一步检查它们的URL是否有效,以及是否有权限访问。
5. **存储数据**:
可以将提取到的数据打印出来,也可以保存到文件或数据库中。这取决于你的具体需求。
6. **注意事项**:
- 遵守网站Robots协议,不要过度频繁地请求数据,以免被封IP。
- 使用代理IP、设置延迟以避免过于明显的行为检测。
- 如果有反爬机制,可能需要使用更复杂的技术,如Session管理、模拟浏览器行为等。
编写爬虫程序,提取“https://www.secoo.com/”网站首页的数据与资源的代码
编写一个简单的Python爬虫,使用requests库来获取网页内容,并使用BeautifulSoup库解析HTML数据,可以从"www.secoo.com"首页提取数据。以下是基础示例:
```python
# 导入需要的库
import requests
from bs4 import BeautifulSoup
# 目标URL
url = "https://www.secoo.com/"
# 发送GET请求并获取页面内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
# 提取特定标签的内容(例如标题、链接等)
title = soup.find('title').text # 获取页面标题
links = [a['href'] for a in soup.select('a')] # 提取所有链接
# 打印提取的信息
print("Title:", title)
print("Links:")
for link in links:
print(link)
else:
print(f"Failed to fetch the page. Status code: {response.status_code}")
#
阅读全文
相关推荐
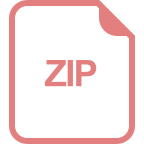
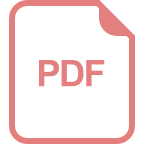
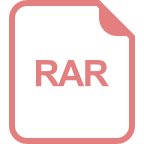
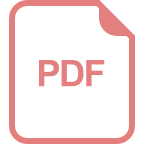
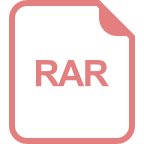
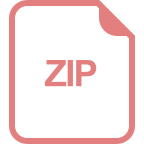
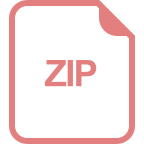
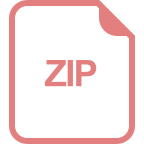
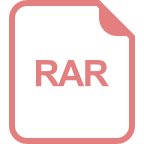
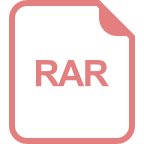
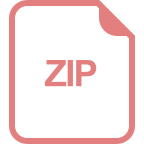
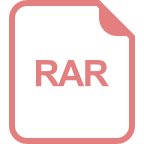