两两交换链表中的节点C语言流程图
时间: 2023-12-24 22:50:30 浏览: 103
以下是交换链表中相邻的两个节点的C语言代码及流程图:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 交换相邻的两个节点
ListNode* swapPairs(ListNode* head) {
ListNode *dummy = (ListNode *)malloc(sizeof(ListNode));
dummy->next = head;
ListNode *cur = dummy;
while (cur->next && cur->next->next) {
ListNode *first = cur->next;
ListNode *second = cur->next->next;
first->next = second->next;
cur->next = second;
cur->next->next = first;
cur = cur->next->next;
}
return dummy->next;
}
// 创建链表
ListNode* createList(int arr[], int n) {
ListNode *dummy = (ListNode *)malloc(sizeof(ListNode));
ListNode *cur = dummy;
for (int i = 0; i < n; i++) {
ListNode *node = (ListNode *)malloc(sizeof(ListNode));
node->val = arr[i];
cur->next = node;
cur = cur->next;
}
cur->next = NULL;
return dummy->next;
}
// 打印链表
void printList(ListNode *head) {
while (head) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(int);
ListNode *head = createList(arr, n);
printf("Original List: ");
printList(head);
head = swapPairs(head);
printf("Swapped List: ");
printList(head);
return 0;
}
```
流程图如下:
```mermaid
graph LR
dummy((dummy)) --> cur((cur))
cur --> |next| node1((1))
node1 --> |next| node2((2))
node2 --> |next| node3((3))
node3 --> |next| node4((4))
node4 --> |next| node5((5))
node5 --> |next| NULL((NULL))
cur --> |next| first((1))
first --> |next| second((2))
second --> |next| node3
cur --> |next| second
second --> |next| first
first --> |next| node4
cur --> |next| first
first --> |next| second
second --> |next| node5
first --> |next| node5
second --> |next| NULL
first --> |next| NULL
dummy --> |next| second
second --> |next| first
first --> |next| node2
second --> |next| node1
first --> |next| node3
second --> |next| node4
first --> |next| node5
second --> |next| NULL
node2 --> |next| node1
node1 --> |next| node4
node4 --> |next| node3
node3 --> |next| node5
node5 --> |next| NULL
```
其中,dummy 表示虚拟头节点,cur 表示当前节点,first 和 second 分别表示交换的两个节点。节点之间的箭头表示它们之间的关系,如 `node1 --> |next| node2` 表示 node1 指向 node2。
阅读全文
相关推荐
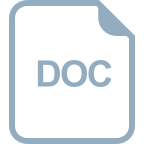
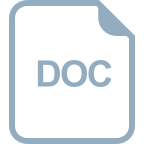
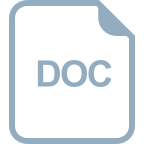
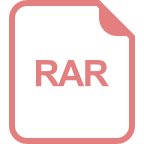
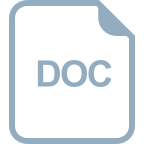
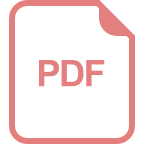
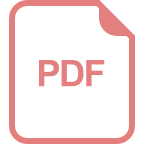
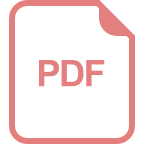
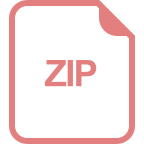
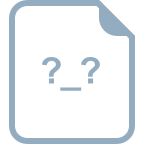
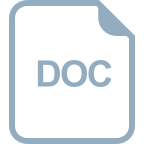
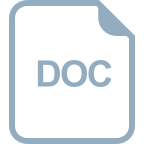
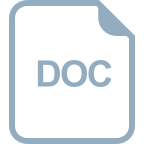
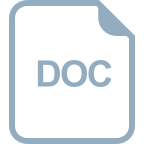
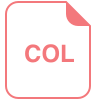
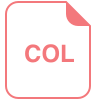


