Let's say we have N modules in a software. A module may use the output of the other modules to complete some tasks. Now, you are provided by an array dep of length N, which stores the dependency of the modules. For example, if module 0 needs the output from module 1 and 2, then dep[0]=[1,2]. Your task is to arrange a proper order for the software to successfully run all the modules one by one. Write a function schedule to return a proper order given the array dep. For example, if dep=[[],[],[0,1]], the proper orders could be [0, 1, 2] or [1, 0, 2], because module 2 depends on module 0 and 1. Note: When there are two modules that can run at the same time, choose the one with smaller node label. Use the above example, both module 0 and 1 should be run before node 2, but you should return [0, 1, 2]. If there is no proper order, return None. For example: Test Result dep = [[],[],[0,1]] print(schedule(dep)) [0, 1, 2]
时间: 2024-03-03 10:49:45 浏览: 12
Sure, I understand your question. Here's a Python function `schedule` that takes an array `dep` and returns a proper order for the software to run all the modules one by one:
```python
def schedule(dep):
n = len(dep)
in_degrees = [0] * n
graph = [[] for _ in range(n)]
for i in range(n):
for j in dep[i]:
in_degrees[i] += 1
graph[j].append(i)
queue = [i for i in range(n) if in_degrees[i] == 0]
res = []
while queue:
node = queue.pop(0)
res.append(node)
for neighbor in graph[node]:
in_degrees[neighbor] -= 1
if in_degrees[neighbor] == 0:
queue.append(neighbor)
return res if len(res) == n else None
```
The function first initializes an `in_degrees` array to store the in-degree of each node in the graph. It then creates an adjacency list `graph` to represent the graph using the `dep` array. The function then initializes a queue with nodes that have in-degree of 0, and processes each node in the queue by adding it to the result and decreasing the in-degree of its neighbors. If the in-degree of a neighbor becomes 0, it is added to the queue. Finally, the function returns the result if the length of the result equals to the number of nodes, otherwise it returns None.
You can test the function with the example you provided:
```python
dep = [[],[],[0,1]]
print(schedule(dep))
```
The output should be `[0, 1, 2]`.
相关推荐
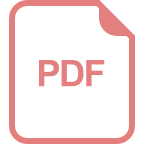
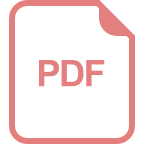
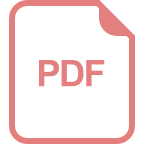














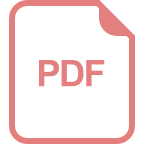