C语言 Windows上监控当前用户访问的网站和IP地址
时间: 2024-06-08 19:09:47 浏览: 175
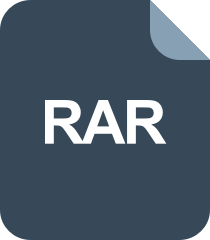
基于windows的网络流量监控

要监控当前用户访问的网站和IP地址,可以通过在Windows上使用WinPcap(Windows Packet Capture)库实现。WinPcap是一个开源的网络数据包捕获库,它允许开发人员捕获和分析网络流量。
以下是一个基本的C语言程序,它使用WinPcap库来监控当前用户访问的网站和IP地址:
```c
#include <stdio.h>
#include <pcap.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
void packet_handler(u_char *param, const struct pcap_pkthdr *header, const u_char *pkt_data);
int main()
{
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t *fp;
int res;
struct pcap_pkthdr *header;
const u_char *pkt_data;
// 打开适配器
fp = pcap_open_live("\\Device\\NPF_{FDD4F9B3-2EDE-45C0-AF97-34D39B79F77D}", 65535, 1, 1000, errbuf);
if (fp == NULL) {
printf("\nUnable to open the adapter. %s is not supported by WinPcap\n", errbuf);
return -1;
}
// 开始捕获数据包
res = pcap_loop(fp, 0, packet_handler, NULL);
if (res == -1) {
printf("Error reading the packets: %s\n", pcap_geterr(fp));
return -1;
}
pcap_close(fp);
return 0;
}
void packet_handler(u_char *param, const struct pcap_pkthdr *header, const u_char *pkt_data)
{
int i = 0;
int tcp_header_length = 0;
const u_char *data;
const u_char *payload;
char src_ip[16];
char dst_ip[16];
int src_port = 0;
int dst_port = 0;
int data_len = 0;
struct ip_header {
u_char ip_header_len : 4; // IP头长度
u_char ip_version : 4; // IP版本号
u_char ip_tos; // 服务类型
u_short ip_total_length; // IP数据包的总长度
u_short ip_id; // 标识
u_short ip_offset; // 分片偏移
u_char ip_ttl; // 存活时间
u_char ip_protocol; // 协议类型
u_short ip_checksum; // 校验和
struct in_addr ip_srcaddr; // 源IP地址
struct in_addr ip_destaddr; // 目的IP地址
} *iph;
struct tcp_header {
u_short source_port; // 源端口号
u_short dest_port; // 目的端口号
u_int sequence; // 序列号
u_int acknowledge; // 确认号
u_char reserved : 4; // 保留
u_char tcp_offset : 4; // TCP头长度
u_char flags; // 标志位
u_short windows; // 窗口大小
u_short checksum; // 校验和
u_short urgent_pointer; // 紧急指针
} *tcph;
iph = (struct ip_header *)(pkt_data + 14); // 以太网头长度为14个字节
tcph = (struct tcp_header *)(pkt_data + 14 + (iph -> ip_header_len * 4)); // TCP头长度为4个字节
// 获取源IP和目的IP地址
strcpy(src_ip, inet_ntoa(iph -> ip_srcaddr));
strcpy(dst_ip, inet_ntoa(iph -> ip_destaddr));
// 获取源端口和目的端口号
src_port = ntohs(tcph -> source_port);
dst_port = ntohs(tcph -> dest_port);
// 获取TCP数据包的数据部分
data = pkt_data + 14 + (iph -> ip_header_len * 4) + (tcph -> tcp_offset * 4);
data_len = ntohs(iph -> ip_total_length) - (iph -> ip_header_len * 4) - (tcph -> tcp_offset * 4);
// 如果是HTTP协议
if (tcph -> dest_port == 80 || tcph -> source_port == 80) {
char *http_data = (char *)data;
int http_len = data_len;
// 查找"Host:"字段
payload = (u_char *)strstr((const char *)http_data, "Host:");
if (payload != NULL) {
payload += 6; // 跳过"Host:"字段
while (isspace(*payload)) payload++; // 跳过空格
// 输出访问的网站和IP地址
printf("Website: %.*s\n", (int)(http_len - (payload - http_data)), payload);
printf("IP Address: %s\n", dst_ip);
}
}
}
```
请注意,上述代码中使用的适配器名称(即\\Device\\NPF_{FDD4F9B3-2EDE-45C0-AF97-34D39B79F77D})是一个实际存在的适配器名称。您需要将其替换为您自己计算机上的适配器名称。可以使用WinPcap库提供的pcap_findalldevs()函数来查找所有可用的适配器名称。
阅读全文
相关推荐
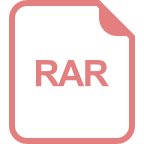
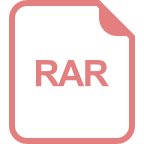
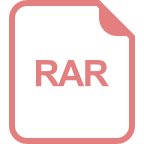
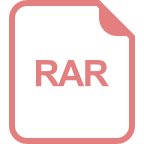
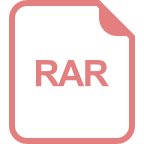
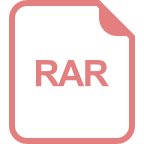
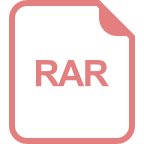
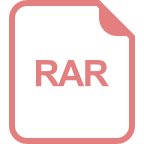
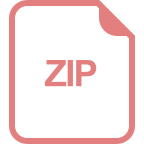
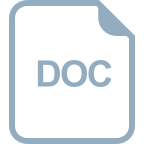
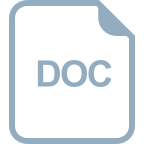
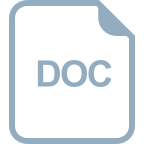
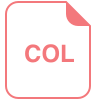
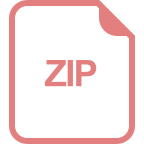
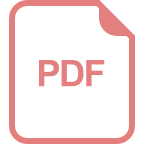