用python 写一个embase 的爬虫
时间: 2023-09-04 11:04:22 浏览: 178
### 回答1:
首先,你需要安装 `requests` 和 `beautifulsoup4` 这两个库。你可以使用以下命令进行安装:
```python
pip install requests beautifulsoup4
```
接下来,你需要了解得到 Embase 文献的方法。这里提供一种使用 Embase 的 API 获取文献的方式。
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头
headers = {
'Content-Type': 'application/json',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 设置请求参数
params = {
"query": "heart disease", # 搜索关键词
"limit": 100, # 搜索结果数量
"offset": 0, # 搜索结果偏移量
"sort": "relevance", # 搜索结果排序方式
"view": "complete", # 搜索结果详细信息
"lang": "en" # 搜索结果语言
}
# 发送请求
response = requests.post('http://api.elsevier.com/content/search/scopus', json=params, headers=headers)
# 解析响应数据
soup = BeautifulSoup(response.text, "html.parser")
for item in soup.find_all('entry'):
# 获取文献标题和作者
title = item.find('dc:title').text
authors = [author.text for author in item.find_all('dc:creator')]
print(title, authors)
```
在这个例子中,我们使用了 Embase 的 API,设置了请求参数,发送了请求,并解析了响应数据。
需要提醒的是,使用 Embase 的 API 需要注册并获得 API Key,这里就不再赘述了。
### 回答2:
为了用Python编写一个简单的embase爬虫,我们可以使用requests和BeautifulSoup库来发送HTTP请求和解析HTML页面。
首先,我们需要安装所需的库。在命令行中使用以下命令安装库:
```
pip install requests beautifulsoup4
```
然后,我们可以开始编写爬虫的代码。下面是一个简单的示例:
```python
import requests
from bs4 import BeautifulSoup
def embase_crawler(query):
# 构建查询URL
url = f"https://www.embase.com/search/results?query={query}"
# 发送HTTP GET请求并获取页面内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析页面内容
soup = BeautifulSoup(response.content, "html.parser")
# 在解析后的页面中查找所需的数据
# 这里以输出搜索结果的标题为例
results = soup.find_all("h3")
for result in results:
print(result.text)
else:
print("请求失败")
# 测试爬虫
query = "python"
embase_crawler(query)
```
在这个例子中,我们定义了一个名为`embase_crawler`的函数,该函数接受一个查询参数。我们使用该查询参数构建embase搜索的URL,并发送GET请求获取页面内容。然后,我们使用BeautifulSoup解析页面,并在解析结果中查找我们需要的数据(这里假设我们搜索结果的标题是在`h3`标签中)。最后,我们打印出搜索结果的标题。
你可以根据自己的需求和embase网站的页面结构来修改代码,以获取其他所需的数据。
### 回答3:
使用Python编写一个embase爬虫可以帮助我们自动获取embase数据库的相关数据。下面是一个基本的爬虫示例代码:
```python
import requests
from bs4 import BeautifulSoup
def embase_crawler():
# 设置搜索关键词
keyword = "example" # 请替换成你想要搜索的关键词
# 构建搜索URL
base_url = "https://www.embase.com/search/"
search_url = base_url + "?quicksearch={}".format(keyword)
# 发送HTTP请求并获取页面内容
response = requests.get(search_url)
if response.status_code == 200:
# 使用BeautifulSoup进行解析
soup = BeautifulSoup(response.text, "html.parser")
# 提取需要的数据
articles = soup.find_all("article")
for article in articles:
title = article.find("h3").text.strip()
authors = article.find("span", class_="result-item_authors").text.strip()
abstract = article.find("div", class_="result-item_abstract").text.strip()
# 打印结果
print("Title: ", title)
print("Authors: ", authors)
print("Abstract: ", abstract)
print("--------------------------------")
else:
print("Failed to retrieve data from embase.")
# 运行爬虫
embase_crawler()
```
注意:上述代码仅为示例,可能无法直接运行,具体要根据实际情况调整和改进。此外,爬虫的使用需要遵守相关网站的使用条款和政策,以免违反相关法律法规。
阅读全文
相关推荐

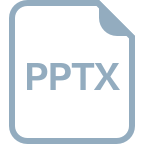
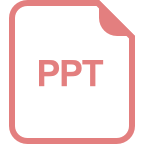
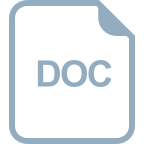
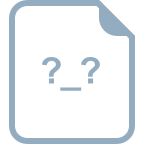
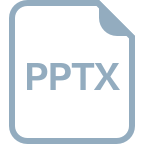
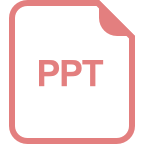
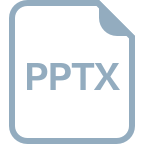
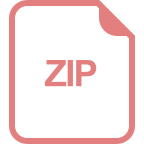
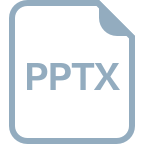
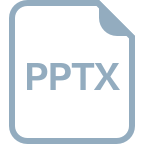
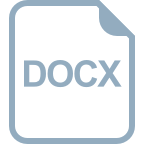
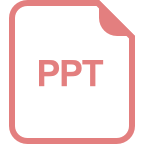