c++ qt tableWidget
时间: 2023-12-01 21:05:09 浏览: 34
A tableWidget in Qt is a widget that displays tabular data in a grid format. It allows users to interactively edit and manipulate data in a tabular form.
To create a tableWidget in Qt, follow these steps:
1. Open Qt Creator and create a new project.
2. Add a tableWidget to your project by dragging it from the widget box to your main window.
3. Set the number of rows and columns in the tableWidget using the setRowCount() and setColumnCount() functions.
4. Populate the tableWidget with data by using the setItem() function to set the contents of each cell.
5. Add any necessary widgets or functions to allow users to interact with the tableWidget, such as adding buttons to add or delete rows, or allowing users to sort the data in the table.
Here's an example of how to create a tableWidget with three columns and five rows:
```
#include <QtWidgets>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QTableWidget tableWidget;
tableWidget.setWindowTitle("My Table Widget");
tableWidget.setRowCount(5);
tableWidget.setColumnCount(3);
// Set the headers for the tableWidget
QStringList headers;
headers << "Name" << "Age" << "Gender";
tableWidget.setHorizontalHeaderLabels(headers);
// Populate the tableWidget with data
QTableWidgetItem *nameItem = new QTableWidgetItem("John");
QTableWidgetItem *ageItem = new QTableWidgetItem("25");
QTableWidgetItem *genderItem = new QTableWidgetItem("Male");
tableWidget.setItem(0, 0, nameItem);
tableWidget.setItem(0, 1, ageItem);
tableWidget.setItem(0, 2, genderItem);
// Show the tableWidget
tableWidget.show();
return app.exec();
}
```
This code creates a tableWidget with three columns ("Name", "Age", and "Gender") and five rows, and populates the first row with data. The setHorizontalHeaderLabels() function sets the headers for the columns. The setItem() function is used to set the contents of each cell in the tableWidget.
You can customize the appearance and behavior of the tableWidget by using various functions and properties. For example, you can set the size of the cells, change the font of the text in the cells, or allow users to edit the contents of the cells.
相关推荐
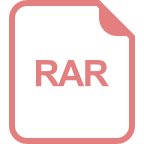
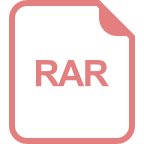














