将查询和统计的结果,当前的数据全部写入文件
时间: 2024-03-10 17:45:35 浏览: 51
要将查询和统计的结果以及当前的数据全部写入文件,可以使用ofstream类来进行文件的写入操作。下面是一个简单的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct Person {
string name;
int age;
string city;
};
int main()
{
vector<Person> people = {
{"Tom", 20, "Beijing"},
{"Jerry", 25, "Shanghai"},
{"Alice", 22, "Guangzhou"},
{"Bob", 28, "Shenzhen"}
};
// 将当前数据写入文件
string filename = "data.txt";
ofstream outfile(filename);
if (outfile.is_open()) {
for (auto p : people) {
outfile << p.name << "," << p.age << "," << p.city << endl;
}
outfile.close();
} else {
cout << "Unable to create file " << filename << endl;
}
// 查询和统计数据
int count = 0;
for (auto p : people) {
if (p.age >= 25) {
cout << p.name << " is older than 25." << endl;
count++;
}
}
// 将查询和统计结果写入文件
ofstream resultfile("result.txt");
if (resultfile.is_open()) {
resultfile << "Number of people older than 25: " << count << endl;
resultfile.close();
} else {
cout << "Unable to create file result.txt" << endl;
}
return 0;
}
```
在上面的代码中,我们首先将当前的数据写入了文件,然后进行了查询和统计操作,并将结果写入了另一个文件。需要注意的是,当写入文件时,我们使用了逗号来分隔不同的字段,在读取文件时需要使用相同的方式进行解析。
阅读全文
相关推荐













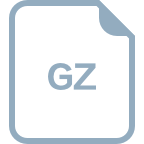
