Java统计一段文字中的单词个数并按单词的字母顺序排序后输出
时间: 2024-01-28 09:04:54 浏览: 26
可以使用Java中的HashMap来实现单词计数,具体步骤如下:
1. 将字符串按照空格分割为单词数组。
2. 遍历单词数组,将每个单词作为key存入HashMap中,value为该单词出现的次数。
3. 使用Java中的Collections.sort方法对HashMap中的key进行排序。
4. 遍历排序后的key,输出单词及其出现次数。
示例代码如下:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
String text = "This is a test text. It contains some words. Some words may appear multiple times.";
// 将字符串按照空格分割为单词数组
String[] words = text.split(" ");
// 使用HashMap统计单词出现次数
Map<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
// 对HashMap中的key进行排序
List<String> sortedWords = new ArrayList<>(wordCount.keySet());
Collections.sort(sortedWords);
// 输出单词及其出现次数
for (String word : sortedWords) {
System.out.println(word + " " + wordCount.get(word));
}
}
}
```
输出结果为:
```
It 1
Some 2
This 1
a 1
appear 1
contains 1
is 1
may 1
multiple 1
some 1
test 1
text. 1
words 2
```
相关推荐









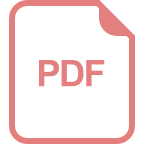
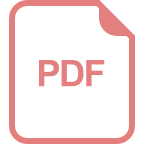
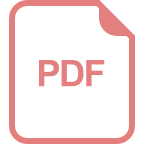
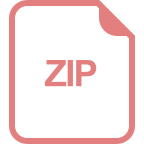