帮我用python设计一个益智类小游戏
时间: 2023-08-31 08:44:12 浏览: 111
### 回答1:
非常感谢您的提问。我可以回答这个问题。关于设计益智类小游戏,Python是一个非常好的选择。您可以使用Python的Pygame库来创建游戏。您可以设计迷宫游戏、拼图游戏、数字游戏等等。具体实现方式可以参考Pygame的官方文档和相关教程。希望我的回答能够帮到您。
### 回答2:
好的,我为您设计一个使用 Python 编写的益智类小游戏。
游戏规则如下:您需要猜一个由程序生成的随机数字,该数字位于1到100之间。您每次可以输入一个数字进行猜测,程序会给出相应的提示,告诉您猜的数字是太大还是太小。您需要继续猜,直到猜中为止。
下面是游戏的代码示例:
```python
import random
def guess_number():
number = random.randint(1, 100) # 生成随机数字
guessed = False # 是否猜中的标志
while not guessed:
guess = int(input("请输入你的猜测数字(1-100):"))
if guess < number:
print("猜的数字太小了!")
elif guess > number:
print("猜的数字太大了!")
else:
print("恭喜,你猜对了!")
guessed = True
guess_number()
```
在这个示例中,我们使用了 Python 的 random 模块来生成一个随机数字,并使用一个 while 循环不断接受用户的输入,进行比较并给出相应的提示,直到用户猜中为止。
这个小游戏可以提高您的猜测能力和数字敏感度,也可以通过更改代码进行扩展,增加计时器、猜测次数统计等功能。希望这个小游戏能带给您一些乐趣和挑战!
### 回答3:
当然可以。以下是一个简单的基于Python的迷宫游戏的设计示例。
```python
import random
# 创建迷宫类
class Maze:
def __init__(self, height, width):
self.height = height
self.width = width
self.maze = [[1] * width for _ in range(height)]
# 打印迷宫
def print_maze(self):
for row in self.maze:
print("".join(["#" if cell == 1 else " " for cell in row]))
# 创建随机迷宫
def generate_maze(self):
stack = [(0, 0)]
self.maze[0][0] = 0
while stack:
current = stack[-1]
neighbors = self.get_neighbors(current)
if neighbors:
next_cell = random.choice(neighbors)
self.remove_wall(current, next_cell)
stack.append(next_cell)
self.maze[next_cell[0]][next_cell[1]] = 0
else:
stack.pop()
# 获取当前位置的相邻可访问位置
def get_neighbors(self, cell):
row, col = cell
neighbors = []
if row > 1 and self.maze[row - 2][col] == 1:
neighbors.append((row - 2, col))
if row < self.height - 2 and self.maze[row + 2][col] == 1:
neighbors.append((row + 2, col))
if col > 1 and self.maze[row][col - 2] == 1:
neighbors.append((row, col - 2))
if col < self.width - 2 and self.maze[row][col + 2] == 1:
neighbors.append((row, col + 2))
return neighbors
# 移除两个位置之间的墙壁
def remove_wall(self, current, next_cell):
row = (current[0] + next_cell[0]) // 2
col = (current[1] + next_cell[1]) // 2
self.maze[row][col] = 0
# 创建游戏类
class Game:
def __init__(self, size):
self.size = size
self.maze = Maze(size * 2 + 1, size * 2 + 1)
self.player = (1, 1)
self.exit = (size * 2 - 1, size * 2 - 1)
self.maze.generate_maze()
# 打印游戏界面
def print_game(self):
for r in range(self.size * 2 + 1):
for c in range(self.size * 2 + 1):
if (r, c) == self.player:
print("P", end=" ")
elif (r, c) == self.exit:
print("E", end=" ")
else:
print("#" if self.maze.maze[r][c] == 1 else " ", end=" ")
print()
# 移动玩家位置
def move_player(self, direction):
row, col = self.player
if direction == "w" and row > 0 and self.maze.maze[row - 1][col] == 0:
self.player = (row - 1, col)
elif direction == "s" and row < self.size * 2 and self.maze.maze[row + 1][col] == 0:
self.player = (row + 1, col)
elif direction == "a" and col > 0 and self.maze.maze[row][col - 1] == 0:
self.player = (row, col - 1)
elif direction == "d" and col < self.size * 2 and self.maze.maze[row][col + 1] == 0:
self.player = (row, col + 1)
if self.player == self.exit:
print("恭喜你成功逃出迷宫!")
# 游戏运行
def main():
size = int(input("请输入迷宫大小(奇数): "))
game = Game(size)
game.print_game()
while True:
direction = input("请输入移动方向(w:上,s:下,a:左,d:右):")
if direction in ("w", "s", "a", "d"):
game.move_player(direction)
game.print_game()
else:
print("请输入合法的方向指令!")
if __name__ == "__main__":
main()
```
这个小游戏是一个迷宫逃脱的游戏,玩家可以使用"w"、"s"、"a"和"d"分别控制角色向上、向下、向左和向右移动,在迷宫中寻找出口。玩家成功逃脱迷宫后游戏结束。在程序中,迷宫的创建使用了随机Prim算法,保证每个位置都可达。迷宫以'#'表示墙壁,以'P'表示角色,以'E'表示出口。
相关推荐
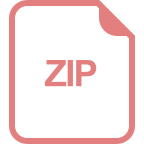
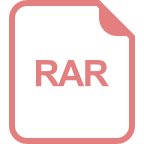
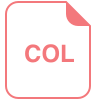













