c++编写一个cal函数,函数作用:计算内存中两个矩阵的乘积 函数的返回值:无
时间: 2023-12-07 19:06:08 浏览: 36
好的,以下是一个简单的C++代码实现:
```c++
void cal(int **matrix1, int **matrix2, int **result, int row1, int col1, int col2) {
for(int i = 0; i < row1; i++) {
for(int j = 0; j < col2; j++) {
int sum = 0;
for(int k = 0; k < col1; k++) {
sum += matrix1[i][k] * matrix2[k][j];
}
result[i][j] = sum;
}
}
}
```
以上代码中,我们通过一个三重循环来计算两个矩阵的乘积,其中 matrix1 和 matrix2 分别为两个待计算矩阵,result 为结果矩阵,row1、col1 和 col2 分别为这三个矩阵的行数和列数。这里我们假设传入的矩阵都是动态申请的二维数组,因此我们使用双指针 int ** 来接收参数。
相关问题
c++编写一个read函数,作用:将文件中的矩阵读入内存的数组中 函数的返回值:读取成功返回0,失败返回-1
下面是一个简单的 C++ 函数,用于从文件中读取矩阵并将其存储到数组中。如果读取成功,函数将返回0,否则返回-1。
```c++
#include <fstream>
#include <vector>
int read_matrix(const char* filename, std::vector<std::vector<int>>& matrix) {
std::ifstream file(filename);
if (!file.is_open()) {
return -1; // 打开文件失败,返回-1
}
matrix.clear(); // 清空矩阵
std::string line;
while (std::getline(file, line)) {
std::vector<int> row;
std::istringstream iss(line);
int num;
while (iss >> num) {
row.push_back(num);
}
if (!row.empty()) {
matrix.push_back(row);
}
}
file.close();
return 0; // 读取成功,返回0
}
```
这个函数接受一个文件名和一个二维向量作为参数,读取文件中的矩阵,并将其存储到二维向量中。每一行表示矩阵中的一行,每一列之间用空格分隔。如果读取成功,函数返回0,否则返回-1。
使用示例:
```c++
#include <iostream>
int main() {
std::vector<std::vector<int>> matrix;
if (read_matrix("matrix.txt", matrix) == 0) {
// 读取成功,输出矩阵
for (const auto& row : matrix) {
for (const auto& num : row) {
std::cout << num << " ";
}
std::cout << std::endl;
}
} else {
// 读取失败,输出错误信息
std::cout << "Failed to read matrix from file" << std::endl;
}
return 0;
}
```
c++编写一个saveResult函数,函数作用:将矩阵相乘的结果存放到文件“result.txt”中 函数的返回值:保存成功返回0,失败返回-1
好的,以下是一个简单的C++代码实现:
```c++
#include <fstream>
int saveResult(int **result, int row, int col) {
std::ofstream outfile("result.txt");
if(!outfile) {
return -1; // 打开文件失败
}
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
outfile << result[i][j] << ' ';
}
outfile << '\n';
}
outfile.close();
return 0; // 保存成功
}
```
以上代码中,我们使用 C++ 的文件流对象 std::ofstream 来打开文件 "result.txt",并将矩阵相乘的结果写入到该文件中。如果打开文件失败,我们返回 -1,表示保存失败;否则,我们返回 0,表示保存成功。
相关推荐
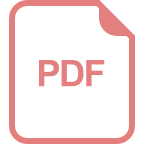
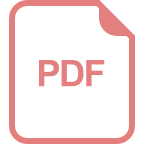












