用java写一个复杂的telnet执行命令
时间: 2023-09-14 19:02:44 浏览: 84
以下是一个使用Java编写的Telnet客户端,它可以连接到远程主机并执行命令:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
import java.net.UnknownHostException;
public class TelnetClient {
private static final String HOST = "localhost";
private static final int PORT = 23;
private static final String USERNAME = "username";
private static final String PASSWORD = "password";
public static void main(String[] args) {
try {
// Connect to the remote host
Socket socket = new Socket(HOST, PORT);
// Get input and output streams for the socket
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
// Wait for the login prompt
String response = in.readLine();
System.out.println(response);
// Send the username
out.println(USERNAME);
response = in.readLine();
System.out.println(response);
// Send the password
out.println(PASSWORD);
response = in.readLine();
System.out.println(response);
// Send a command
out.println("ls");
response = in.readLine();
while (response != null) {
System.out.println(response);
response = in.readLine();
}
// Close the streams and socket
in.close();
out.close();
socket.close();
} catch (UnknownHostException e) {
System.err.println("Unknown host: " + HOST);
} catch (IOException e) {
System.err.println("I/O error: " + e.getMessage());
}
}
}
```
在这个例子中,我们使用了Java标准库中的Socket类来连接到远程主机。我们使用BufferedReader和PrintWriter类来读写数据。我们首先等待登录提示,然后发送用户名和密码。然后我们发送一个命令(这里是“ls”),并读取输出直到结束。最后,我们关闭输入和输出流以及套接字。请注意,这个例子只是一个简单的示例,实际应用程序需要更多的错误处理和安全性检查。
阅读全文
相关推荐
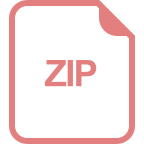
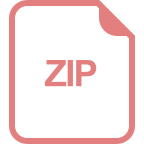
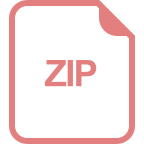
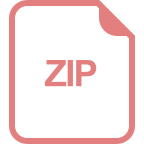
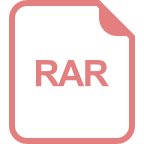
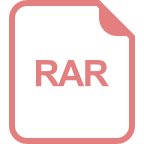








