data = ds.variables['variable_name'][:]里的['variable_name 要填什么
时间: 2023-10-06 07:13:06 浏览: 41
在 `data = ds.variables['variable_name'][:]` 这行代码中,`'variable_name'` 是 NetCDF 文件中变量的名称,你需要将其替换为你实际要读取的变量名称。
在 NetCDF 文件中,变量可以是标量、一维数组、二维数组或更高维数组,它们都有一个唯一的名称。你可以在 NetCDF 文件中使用 `ncdump` 命令或者 `netCDF4` 库来查看变量的名称。
例如,假设你要读取名为 `'temperature'` 的变量,那么代码应该是这样的:
```python
import netCDF4
# 打开 NetCDF 文件
nc_file = netCDF4.Dataset('file_path.nc', 'r')
# 读取名为 'temperature' 的变量
data = nc_file.variables['temperature'][:]
# 关闭 NetCDF 文件
nc_file.close()
```
在上面的示例代码中,我们使用 `nc_file.variables['temperature']` 来获取名为 `'temperature'` 的变量,并使用 `[:]` 语法来读取该变量的全部数据。最后,我们使用 `close()` 方法关闭 NetCDF 文件。
相关问题
if "__main__" == __name__:
If "__main__" == __name__:, it is a conditional statement commonly used in Python scripts. It checks if the current module is being run directly as the main program. In other words, it ensures that certain code is only executed when the module is run directly and not when it is imported as a module in another script.
This check is useful when you have some code that should only run when the script is executed directly, such as initializing variables, defining functions, or running specific operations. When the module is imported, this code block will be skipped.
Here's an example to illustrate its usage:
```python
def main():
# code to be executed when the script is run directly
pass
if "__main__" == __name__:
main()
```
In this example, the `main()` function will only be executed if the script is run directly. If the module is imported by another script, the `main()` function will not be executed.
import time import tensorflow.compat.v1 as tf tf.disable_v2_behavior() from tensorflow.examples.tutorials.mnist import input_data import mnist_inference import mnist_train tf.compat.v1.reset_default_graph() EVAL_INTERVAL_SECS = 10 def evaluate(mnist): with tf.Graph().as_default() as g: #定义输入与输出的格式 x = tf.compat.v1.placeholder(tf.float32, [None, mnist_inference.INPUT_NODE], name='x-input') y_ = tf.compat.v1.placeholder(tf.float32, [None, mnist_inference.OUTPUT_NODE], name='y-input') validate_feed = {x: mnist.validation.images, y_: mnist.validation.labels} #直接调用封装好的函数来计算前向传播的结果 y = mnist_inference.inference(x, None) #计算正确率 correcgt_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1)) accuracy = tf.reduce_mean(tf.cast(correcgt_prediction, tf.float32)) #通过变量重命名的方式加载模型 variable_averages = tf.train.ExponentialMovingAverage(0.99) variable_to_restore = variable_averages.variables_to_restore() saver = tf.train.Saver(variable_to_restore) #每隔10秒调用一次计算正确率的过程以检测训练过程中正确率的变化 while True: with tf.compat.v1.Session() as sess: ckpt = tf.train.get_checkpoint_state(minist_train.MODEL_SAVE_PATH) if ckpt and ckpt.model_checkpoint_path: #load the model saver.restore(sess, ckpt.model_checkpoint_path) global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1] accuracy_score = sess.run(accuracy, feed_dict=validate_feed) print("After %s training steps, validation accuracy = %g" % (global_step, accuracy_score)) else: print('No checkpoint file found') return time.sleep(EVAL_INTERVAL_SECS) def main(argv=None): mnist = input_data.read_data_sets(r"D:\Anaconda123\Lib\site-packages\tensorboard\mnist", one_hot=True) evaluate(mnist) if __name__ == '__main__': tf.compat.v1.app.run()对代码进行改进
以下是对代码的改进建议:
1. 在代码开头添加注释,简要说明代码功能和使用方法。
2. 将导入模块的语句放在代码开头。
3. 将模型保存路径和评估时间间隔定义为常量,并使用有意义的变量名。
4. 将计算正确率和加载模型的过程封装为函数。
5. 在主函数中调用评估函数。
改进后的代码如下:
```
# 该代码实现了使用已训练好的模型对 MNIST 数据集进行评估
import time
import tensorflow.compat.v1 as tf
from tensorflow.examples.tutorials.mnist import input_data
import mnist_inference
import mnist_train
# 定义常量
MODEL_SAVE_PATH = 'model/'
EVAL_INTERVAL_SECS = 10
def evaluate(mnist):
"""
计算模型在验证集上的正确率
"""
with tf.Graph().as_default() as g:
# 定义输入和输出格式
x = tf.placeholder(tf.float32, [None, mnist_inference.INPUT_NODE], name='x-input')
y_ = tf.placeholder(tf.float32, [None, mnist_inference.OUTPUT_NODE], name='y-input')
# 直接调用封装好的函数计算前向传播结果
y = mnist_inference.inference(x, None)
# 计算正确率
correct_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 加载模型
variable_averages = tf.train.ExponentialMovingAverage(mnist_train.MOVING_AVERAGE_DECAY)
variables_to_restore = variable_averages.variables_to_restore()
saver = tf.train.Saver(variables_to_restore)
# 在验证集上计算正确率
with tf.Session() as sess:
ckpt = tf.train.get_checkpoint_state(MODEL_SAVE_PATH)
if ckpt and ckpt.model_checkpoint_path:
saver.restore(sess, ckpt.model_checkpoint_path)
global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1]
accuracy_score = sess.run(accuracy, feed_dict={x: mnist.validation.images, y_: mnist.validation.labels})
print("After %s training steps, validation accuracy = %g" % (global_step, accuracy_score))
else:
print('No checkpoint file found')
def main(argv=None):
# 读取数据集
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
# 每隔一定时间评估模型在验证集上的正确率
while True:
evaluate(mnist)
time.sleep(EVAL_INTERVAL_SECS)
if __name__ == '__main__':
tf.app.run()
```
相关推荐
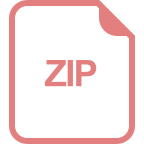
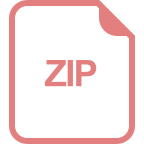
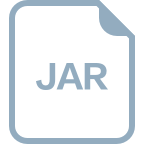
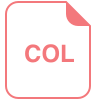
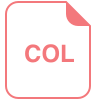











