C++有存放username的名为saving文本文件,现在每次输入一个不重复username可以在MySQL创建与username对应的表并能对表进行增删查改的操作
时间: 2024-02-24 17:57:39 浏览: 62
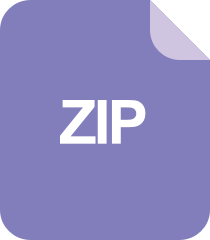
通过API函数对Mysql数据库实现增、删、改、查操作C++实现
好的,我会尽力回答您的问题。
首先,您需要读取`saving`文件中的用户名,并将其存储到一个`vector<string>`中,以便后续使用。这可以通过以下代码实现:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
int main() {
vector<string> usernames;
string username;
ifstream fin("saving.txt");
while (fin >> username) {
usernames.push_back(username);
}
fin.close();
// 现在usernames中存储了所有的用户名
return 0;
}
```
接下来,您需要连接到MySQL数据库。这可以通过使用MySQL C++ Connector库实现。您可以从MySQL官网下载并安装这个库。在连接数据库之前,您需要先创建一个数据库和一个用户名和密码,以便于连接。假设您的数据库名为`mydb`,用户名为`myuser`,密码为`mypassword`,则可以通过以下代码连接到MySQL数据库:
```c++
#include <iostream>
#include <string>
#include <mysql/mysql.h>
using namespace std;
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, "localhost", "myuser", "mypassword", "mydb", 0, NULL, 0)) {
cout << "Error connecting to MySQL: " << mysql_error(conn) << endl;
return 1;
}
// 现在已经成功连接到MySQL数据库
return 0;
}
```
现在,您已经成功连接到MySQL数据库,接下来您需要创建一个表,以便于存储每个用户名对应的数据。假设您的表名为`mytable`,包含两个字段`name`和`data`,则可以通过以下代码创建这个表:
```c++
#include <iostream>
#include <string>
#include <mysql/mysql.h>
using namespace std;
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, "localhost", "myuser", "mypassword", "mydb", 0, NULL, 0)) {
cout << "Error connecting to MySQL: " << mysql_error(conn) << endl;
return 1;
}
if (mysql_query(conn, "CREATE TABLE mytable (name VARCHAR(50), data TEXT)")) {
cout << "Error creating table: " << mysql_error(conn) << endl;
return 1;
}
// 现在已经成功创建了一个名为mytable的表
return 0;
}
```
接下来,您需要实现增删查改的操作。假设您已经从`saving`文件中读取了所有的用户名并存储在`usernames`中,您可以通过以下代码实现向`mytable`表中插入数据的操作:
```c++
#include <iostream>
#include <string>
#include <mysql/mysql.h>
using namespace std;
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, "localhost", "myuser", "mypassword", "mydb", 0, NULL, 0)) {
cout << "Error connecting to MySQL: " << mysql_error(conn) << endl;
return 1;
}
for (int i = 0; i < usernames.size(); i++) {
string username = usernames[i];
string data = "some data for " + username;
string query = "INSERT INTO mytable (name, data) VALUES ('" + username + "', '" + data + "')";
if (mysql_query(conn, query.c_str())) {
cout << "Error inserting data: " << mysql_error(conn) << endl;
return 1;
}
}
// 现在已经成功向mytable表中插入了数据
return 0;
}
```
同样地,您可以使用类似的代码实现删除、查询和修改的操作。例如,以下代码实现了根据用户名删除表中数据的操作:
```c++
#include <iostream>
#include <string>
#include <mysql/mysql.h>
using namespace std;
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, "localhost", "myuser", "mypassword", "mydb", 0, NULL, 0)) {
cout << "Error connecting to MySQL: " << mysql_error(conn) << endl;
return 1;
}
for (int i = 0; i < usernames.size(); i++) {
string username = usernames[i];
string query = "DELETE FROM mytable WHERE name = '" + username + "'";
if (mysql_query(conn, query.c_str())) {
cout << "Error deleting data: " << mysql_error(conn) << endl;
return 1;
}
}
// 现在已经成功从mytable表中删除了数据
return 0;
}
```
请注意,这里的代码仅供参考,实际操作中还需要考虑许多其他因素,例如错误处理、数据类型转换等。
阅读全文
相关推荐
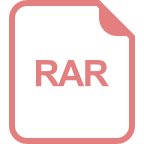
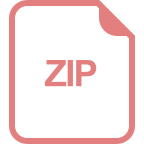
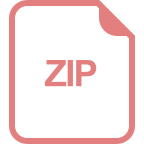
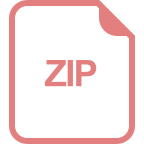
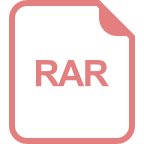
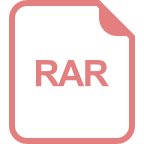
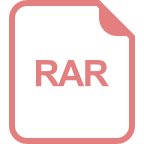
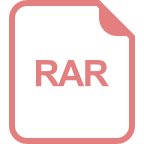
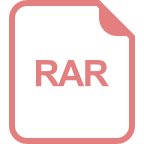
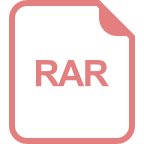
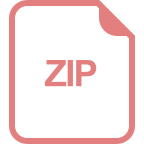
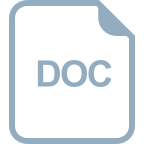
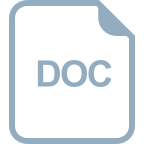
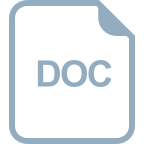
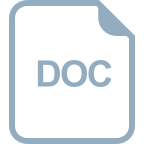