使用python多线程通过网易云API批量爬取热门歌曲基本信息,并存储为csv文件
时间: 2024-05-15 12:16:59 浏览: 174
以下是一个使用Python多线程通过网易云API批量爬取热门歌曲基本信息,并存储为csv文件的示例代码:
```python
import requests
import csv
import threading
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 定义线程锁
lock = threading.Lock()
# 定义一个函数,用于获取热门歌曲的基本信息
def get_song_info(limit, offset):
url = 'https://api.imjad.cn/cloudmusic/?type=topPlayList&cat=%E5%85%A8%E9%83%A8&limit={}&offset={}'.format(
limit, offset)
response = requests.get(url, headers=headers)
data = response.json()
song_list = data['playlists']
for song in song_list:
# 获取歌曲基本信息
song_id = song['id']
song_name = song['name']
song_author = song['creator']['nickname']
song_url = 'https://music.163.com/#/playlist?id={}'.format(song_id)
# 写入csv文件
with lock:
with open('song_info.csv', 'a', encoding='utf-8', newline='') as f:
writer = csv.writer(f)
writer.writerow([song_id, song_name, song_author, song_url])
# 定义一个函数,用于创建并启动多线程
def main():
# 设置热门歌单总数和每页歌单数
total = 100
limit = 35
# 计算需要的线程数
thread_num = total // limit + 1
threads = []
# 创建多个线程
for i in range(thread_num):
offset = i * limit
t = threading.Thread(target=get_song_info, args=(limit, offset))
threads.append(t)
# 启动所有线程
for t in threads:
t.start()
# 等待所有线程执行完成
for t in threads:
t.join()
if __name__ == '__main__':
# 执行主函数
main()
```
该代码使用了Python的requests库发起HTTP请求,并通过网易云音乐API获取热门歌曲的基本信息。使用了多线程将获取歌曲信息的任务分配给多个线程并行执行,提高了爬取速度。最终将获取到的歌曲信息保存为csv文件。
阅读全文
相关推荐
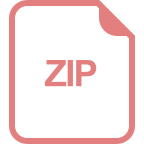
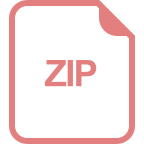
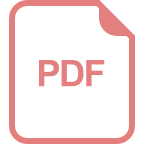
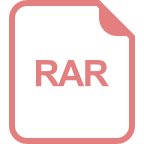
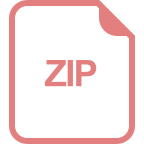
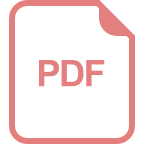
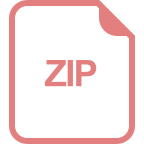
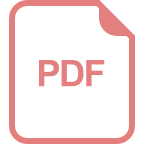
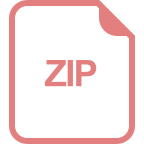
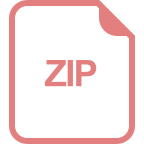
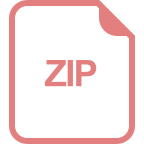
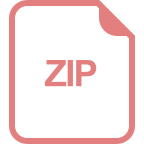
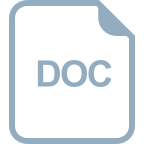