请帮我写一个jsp页面,用于局域网内的浏览器访问mysql数据库,并将查询结果显示在浏览器中。具体需求如下:当从局域网内的浏览器打开时,允许用户输入SN,modeCode,lotNum,sterDate,productMode信息,并提交给服务器,服务器从mysql数据库(数据库名称mysql,表名称table1,IP 192.168.5.100,密码mypassword,端口号默认)的表table1中查询出包含上述输入条件的记录,并显示给客户端。数据库中表table1的字段名包含客户端的输入字段。结果显示时,将数据库的字段名按照下列对应关系展示在浏览器中。SN对应'序列号',modeCode对应'型号',logNum对应'批号',sterDate对应'灭菌日期'。 背景:web服务器为tomcat;使用jdbc数据连接。
时间: 2024-02-09 22:08:10 浏览: 78
好的,以下是一个简单的jsp页面,用于实现你的需求。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>查询结果</title>
</head>
<body>
<h1>查询结果</h1>
<form method="post" action="query.jsp">
<label>SN:</label>
<input type="text" name="sn"><br>
<label>modeCode:</label>
<input type="text" name="modeCode"><br>
<label>lotNum:</label>
<input type="text" name="lotNum"><br>
<label>sterDate:</label>
<input type="date" name="sterDate"><br>
<label>productMode:</label>
<input type="text" name="productMode"><br>
<input type="submit" value="查询">
</form>
<hr>
<%
String sn = request.getParameter("sn");
String modeCode = request.getParameter("modeCode");
String lotNum = request.getParameter("lotNum");
String sterDate = request.getParameter("sterDate");
String productMode = request.getParameter("productMode");
if (sn != null || modeCode != null || lotNum != null || sterDate != null || productMode != null) {
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://192.168.5.100:3306/mysql?useSSL=false";
String username = "root";
String password = "mypassword";
Connection conn = DriverManager.getConnection(url, username, password);
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM table1 WHERE SN='" + sn + "'";
if (modeCode != null) {
sql += " AND modeCode='" + modeCode + "'";
}
if (lotNum != null) {
sql += " AND logNum='" + lotNum + "'";
}
if (sterDate != null) {
sql += " AND sterDate='" + sterDate + "'";
}
if (productMode != null) {
sql += " AND productMode='" + productMode + "'";
}
ResultSet rs = stmt.executeQuery(sql);
out.println("<table border=\"1\">");
out.println("<tr><th>序列号</th><th>型号</th><th>批号</th><th>灭菌日期</th></tr>");
while (rs.next()) {
out.println("<tr>");
out.println("<td>" + rs.getString("SN") + "</td>");
out.println("<td>" + rs.getString("modeCode") + "</td>");
out.println("<td>" + rs.getString("logNum") + "</td>");
out.println("<td>" + rs.getString("sterDate") + "</td>");
out.println("</tr>");
}
out.println("</table>");
rs.close();
stmt.close();
conn.close();
} catch (ClassNotFoundException e) {
out.println("ClassNotFoundException: " + e.getMessage());
} catch (SQLException e) {
out.println("SQLException: " + e.getMessage());
}
}
%>
</body>
</html>
```
这个页面包含一个查询表单,用户可以输入查询条件并提交查询。在服务器端,我们使用jdbc连接到mysql数据库,根据用户输入的条件进行查询,然后将结果显示在表格中。
注意,为了安全起见,这个页面没有进行任何输入验证和SQL注入防护。如果需要在生产环境中使用,你需要进一步加强安全性。
阅读全文
相关推荐









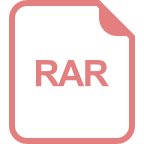








