使用vue3+antd+ts创建一个弹窗组件的代码
时间: 2023-09-10 22:05:42 浏览: 273
### 回答1:
在Vue3中使用Antd和TypeScript创建一个弹窗组件的代码如下:
```html
<template>
<div>
<antd-popover
trigger="click"
title="弹窗标题"
:visible="visible"
@visible-change="handleVisibleChange"
>
<p>弹窗内容</p>
<div slot="action">
<antd-button @click="handleOk">确定</antd-button>
</div>
</antd-popover>
</div>
</template><script lang="ts">
import { Component, Vue, Prop } from 'vue-property-decorator';
import { Popover, Button } from 'ant-design-vue';@Component
export default class PopoverExample extends Vue {
@Prop() visible!: boolean; handleVisibleChange(visible: boolean) {
this.$emit('visible-change', visible);
} handleOk() {
this.$emit('ok');
} render() {
return (
<div>
<Popover
trigger="click"
title="弹窗标题"
visible={this.visible}
onVisibleChange={this.handleVisibleChange}
>
<p>弹窗内容</p>
<div slot="action">
<Button onClick={this.handleOk}>确定</Button>
</div>
</Popover>
</div>
);
}
}
</script>
```
### 回答2:
以下是使用Vue3、Ant Design和TypeScript创建一个弹窗组件的代码示例:
```html
<template>
<a-button type="primary" @click="showModal">打开弹窗</a-button>
<a-modal v-model:visible="visible" title="示例弹窗">
<p>这是一个弹窗的内容。</p>
<p>可根据实际需要自定义弹窗的内容。</p>
<span slot="footer">
<a-button @click="visible = false">关闭</a-button>
<a-button type="primary" @click="handleOk">确定</a-button>
</span>
</a-modal>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
import { Button, Modal } from 'ant-design-vue';
export default defineComponent({
components: {
'a-button': Button,
'a-modal': Modal,
},
setup() {
const visible = ref(false);
const showModal = () => {
visible.value = true;
};
const handleOk = () => {
// 处理确定按钮的逻辑
visible.value = false;
};
return {
visible,
showModal,
handleOk,
};
},
});
</script>
```
在上述代码中,我们使用了Vue3的`defineComponent`方法定义了一个组件,引入了`Button`和`Modal`组件,分别对应Ant Design中的按钮和弹窗。通过在`setup`函数中返回数据和方法,实现了打开弹窗、关闭弹窗和处理确定按钮逻辑的功能。在模板中,使用了`v-model`来绑定弹窗的可见性,通过点击按钮触发`showModal`方法显示弹窗,点击确定按钮触发`handleOk`方法。
### 回答3:
下面是使用Vue 3、Ant Design和Typescript创建弹窗组件的代码示例:
```html
<template>
<a-button type="primary" @click="showModal">打开弹窗</a-button>
<a-modal v-model:visible="isVisible" title="弹窗标题" @ok="handleOk" @cancel="handleCancel">
<p>这是弹窗的内容</p>
</a-modal>
</template>
<script lang="ts">
import { defineComponent, reactive } from 'vue';
import { Button, Modal } from 'ant-design-vue';
export default defineComponent({
components: {
'a-button': Button,
'a-modal': Modal,
},
setup() {
const isVisible = reactive(false);
const showModal = () => {
isVisible.value = true;
};
const handleOk = () => {
isVisible.value = false;
};
const handleCancel = () => {
isVisible.value = false;
};
return {
isVisible,
showModal,
handleOk,
handleCancel
};
},
});
</script>
```
在上述代码中,我们首先使用Vue的`defineComponent`方法定义一个组件。然后引入了`Button`和`Modal`组件,并在`components`选项中注册它们。
在`setup`方法中,我们使用`reactive`函数创建了一个响应式变量`isVisible`来控制弹窗的显示与隐藏。然后定义了`showModal`、`handleOk`和`handleCancel`方法来处理弹窗的打开、确认和取消事件。
在模板中,我们使用`Button`组件创建了一个打开弹窗的按钮,并使用`Modal`组件来创建弹窗。通过`v-model:visible`绑定`isVisible`变量来控制弹窗的显示与隐藏。弹窗的标题为"弹窗标题",并在弹窗内容中添加了一个段落。
当点击"打开弹窗"按钮时,会调用`showModal`方法打开弹窗。弹窗中的确认和取消按钮分别绑定了`handleOk`和`handleCancel`方法。
这样就完成了使用Vue 3、Ant Design和Typescript创建一个弹窗组件的代码。
阅读全文
相关推荐
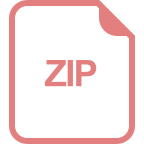
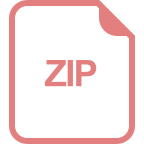
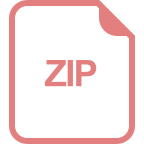
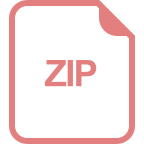
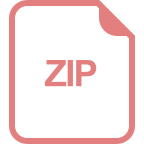
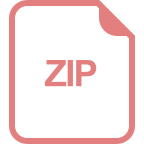
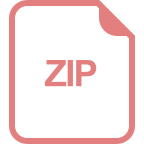
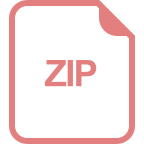
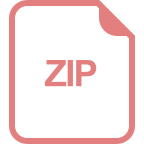
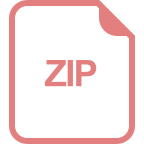
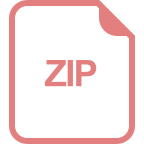
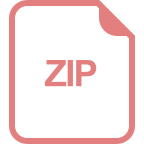
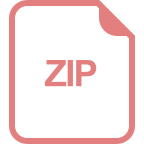
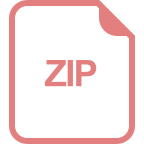
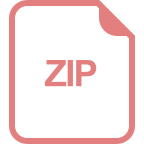
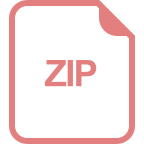