.Net Core Web Api 配置开发PELCO-D/P
时间: 2024-03-15 09:47:01 浏览: 63
在 .Net Core Web Api 中配置 PELCO-D/P 控制功能,需要进行以下步骤:
1. 在项目中添加 PELCO-D/P 相关的依赖,可以使用 NuGet 包管理器添加 SerialPort 或 Socket 相关的依赖。
2. 在 appsettings.json 或 appsettings.Development.json 文件中添加 PELCO-D/P 相关的配置信息,比如串口号、波特率、网络地址、端口号等。
3. 在 Startup.cs 文件中添加 PELCO-D/P 相关的服务和中间件。
4. 在 PelcoController 中读取配置信息,并使用配置信息来初始化串口或网络通信。
5. 在 POST 方法中使用配置信息来解析 PELCO-D/P 指令,并生成协议数据包发送给摄像头。
6. 配置 HTTPS 或其他安全机制,保证控制接口的安全性。
具体的配置方式可以参考以下示例代码:
```csharp
// appsettings.json 文件中配置 PELCO-D/P 相关的信息
{
"PelcoD": {
"PortName": "COM1",
"BaudRate": 9600,
"IPAddress": "192.168.1.100",
"Port": 6001
}
}
// Startup.cs 文件中添加 PELCO-D/P 相关的服务和中间件
public void ConfigureServices(IServiceCollection services)
{
// 注册 PELCO-D/P 相关的服务
services.AddSingleton<PelcoDService>();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// 使用 PELCO-D/P 相关的中间件
app.UseMiddleware<PelcoDMiddleware>();
}
// PelcoController 中读取配置信息,并使用配置信息初始化串口或网络通信
public class PelcoController : Controller
{
private readonly PelcoDService _pelcoDService;
public PelcoController(IConfiguration configuration, PelcoDService pelcoDService)
{
_pelcoDService = pelcoDService;
var pelcoDSettings = configuration.GetSection("PelcoD");
var portName = pelcoDSettings.GetValue<string>("PortName");
var baudRate = pelcoDSettings.GetValue<int>("BaudRate");
var ipAddress = pelcoDSettings.GetValue<string>("IPAddress");
var port = pelcoDSettings.GetValue<int>("Port");
_pelcoDService.Connect(portName, baudRate, ipAddress, port);
}
// POST 方法中解析 PELCO-D/P 指令,并发送给摄像头
[HttpPost]
public IActionResult ControlPelcoD([FromBody] PelcoDCommand command)
{
var data = PelcoDHelper.GenerateCommandData(command);
_pelcoDService.Send(data);
var response = _pelcoDService.Receive();
return Ok(response);
}
}
```
上述代码中,PelcoDService 是一个 PELCO-D/P 通信服务,负责连接、发送和接收数据。PelcoDMiddleware 是一个 PELCO-D/P 中间件,用于验证控制接口的安全性。PelcoDHelper 是一个 PELCO-D/P 辅助类,用于生成协议数据包。
阅读全文
相关推荐
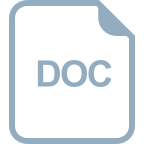
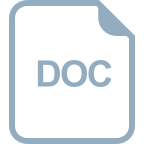
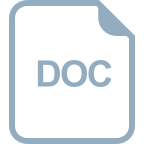

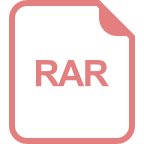
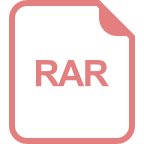
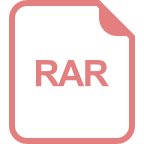
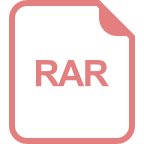
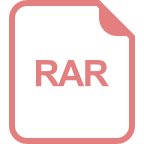
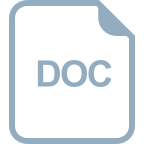
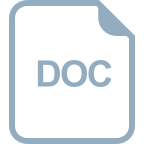
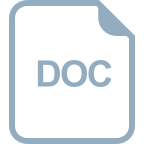
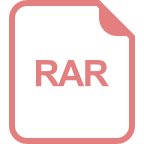
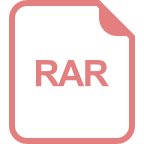

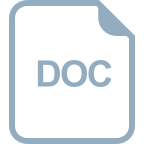