C语言实现 •用户输入一十进制整数,将其转换成对应的二进制、八进制或十六进制数并显示; •用户输入一个算术表达式,包含十、一、*、人、(、)等运算符,系统能分别有效识别操作数和运算符;。 •建立两个栈:操作数栈(OPND)和远算符栈(OPTR),分别将算术表达式中的操作数和运算符进行压栈存储和出栈运算; •确定运算符的优先级,并根据优先级关系进行运算,并输出最终结结果
时间: 2024-01-31 21:02:20 浏览: 28
好的,以下是使用C语言实现的代码实现您的需求:
1. 将十进制整数转换成二进制、八进制或十六进制数
```c
#include <stdio.h>
// 将十进制整数num转换为base进制数
void convert(int num, int base) {
char SYMBOLS[] = "0123456789ABCDEF";
int stack[100], top = -1;
while (num > 0) {
int rem = num % base;
stack[++top] = rem;
num /= base;
}
printf("转换结果为:");
while (top >= 0) {
printf("%c", SYMBOLS[stack[top--]]);
}
printf("\n");
}
int main() {
int num, base;
printf("请输入一个十进制整数:");
scanf("%d", &num);
printf("请输入转换成的进制数(2/8/16):");
scanf("%d", &base);
if (base == 2 || base == 8 || base == 16) {
convert(num, base);
} else {
printf("无效的进制数!\n");
}
return 0;
}
```
2. 对算术表达式进行求值
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 判断是否为运算符
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '(' || c == ')';
}
// 判断运算符优先级
int priority(char op) {
if (op == '*' || op == '/') {
return 2;
} else if (op == '+' || op == '-') {
return 1;
} else {
return 0;
}
}
// 计算表达式
double calculate(char expr[]) {
double OPND[MAX_SIZE]; // 操作数栈
char OPTR[MAX_SIZE]; // 运算符栈
int top1 = -1, top2 = -1;
int i = 0;
while (expr[i] != '\0') {
char c = expr[i];
if (c == ' ') { // 忽略空格
i++;
} else if (!is_operator(c)) { // 操作数
char num[100];
int j = i;
while (expr[j] != '\0' && !is_operator(expr[j])) {
num[j - i] = expr[j];
j++;
}
num[j - i] = '\0';
OPND[++top1] = atof(num);
i = j;
} else { // 运算符
if (top2 == -1 || OPTR[top2] == '(' || priority(c) > priority(OPTR[top2])) {
OPTR[++top2] = c;
i++;
} else if (priority(c) <= priority(OPTR[top2])) {
char op = OPTR[top2--];
double b = OPND[top1--];
double a = OPND[top1--];
double res;
switch (op) {
case '+':
res = a + b;
break;
case '-':
res = a - b;
break;
case '*':
res = a * b;
break;
case '/':
res = a / b;
break;
}
OPND[++top1] = res;
} else if (c == ')') {
top2--;
i++;
}
}
}
while (top2 != -1) {
char op = OPTR[top2--];
double b = OPND[top1--];
double a = OPND[top1--];
double res;
switch (op) {
case '+':
res = a + b;
break;
case '-':
res = a - b;
break;
case '*':
res = a * b;
break;
case '/':
res = a / b;
break;
}
OPND[++top1] = res;
}
return OPND[0];
}
int main() {
char expr[100];
printf("请输入一个算术表达式:");
gets(expr);
double res = calculate(expr);
printf("计算结果为:%f\n", res);
return 0;
}
```
需要注意的是,在使用gets函数接收输入时需要注意输入的字符串长度不要超过数组的最大长度,否则会导致程序崩溃。另外,在输入算术表达式时需要注意空格的处理,以便正确地识别出操作数和运算符。
相关推荐
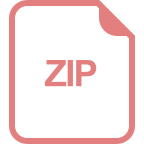














