用java写一个堆排序
时间: 2023-01-28 12:02:27 浏览: 73
可以使用 Java 的 PriorityQueue 类来实现堆排序。这是一个封装好的堆数据结构,默认是小根堆,可以通过传递自定义的 Comparator 实现大根堆。
以下是一个小根堆的示例代码:
```
import java.util.PriorityQueue;
public class HeapSort {
public static void main(String[] args) {
int[] nums = { 3, 5, 2, 1, 4 };
// 使用小根堆进行排序
PriorityQueue<Integer> heap = new PriorityQueue<>();
for (int num : nums) {
heap.offer(num);
}
// 从堆中依次取出最小元素,并输出
while (!heap.isEmpty()) {
System.out.print(heap.poll() + " ");
}
}
}
```
输出结果为:1 2 3 4 5
如果要实现大根堆,可以使用以下代码:
```
import java.util.PriorityQueue;
import java.util.Comparator;
public class HeapSort {
public static void main(String[] args) {
int[] nums = { 3, 5, 2, 1, 4 };
// 使用大根堆进行排序
PriorityQueue<Integer> heap = new PriorityQueue<>(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2 - o1;
}
});
for (int num : nums) {
heap.offer(num);
}
// 从堆中依次取出最大元素,并输出
while (!heap.isEmpty()) {
System.out.print(heap.poll() + " ");
}
}
}
```
输出结果为:5 4 3 2 1
阅读全文
相关推荐
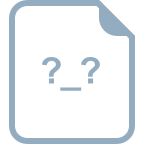
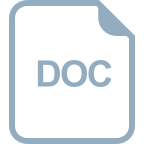
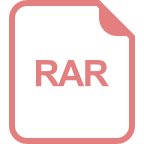
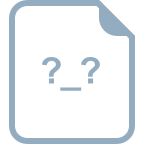
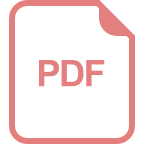
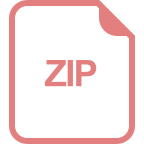
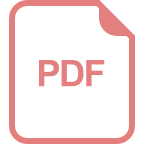
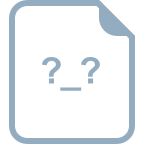
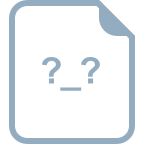