用c++编程一个功能齐全的数独游戏
时间: 2024-05-17 16:13:44 浏览: 118
以下是一个基于 C++ 的数独游戏代码,包含生成数独题目、解题算法、用户界面等功能。代码中使用了回溯法求解数独,生成数独题目使用了随机填数算法。
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <vector>
using namespace std;
// 定义数独的数据结构
const int N = 9;
int board[N][N];
// 打印数独
void print_board() {
cout << "-------------------------" << endl;
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (j % 3 == 0) cout << "| ";
cout << board[i][j] << " ";
}
cout << "|" << endl;
if (i % 3 == 2) cout << "-------------------------" << endl;
}
}
// 检查数字 num 是否可以填入行 row,列 col,九宫格
bool check_num(int num, int row, int col) {
// 检查行和列
for (int i = 0; i < N; i++) {
if (board[row][i] == num || board[i][col] == num) {
return false;
}
}
// 检查九宫格
int row_start = (row / 3) * 3;
int col_start = (col / 3) * 3;
for (int i = row_start; i < row_start + 3; i++) {
for (int j = col_start; j < col_start + 3; j++) {
if (board[i][j] == num) {
return false;
}
}
}
return true;
}
// 回溯法填数
bool solve_board(int row, int col) {
if (row == N) return true;
if (col == N) return solve_board(row + 1, 0);
if (board[row][col] != 0) return solve_board(row, col + 1);
for (int num = 1; num <= 9; num++) {
if (check_num(num, row, col)) {
board[row][col] = num;
if (solve_board(row, col + 1)) {
return true;
}
board[row][col] = 0;
}
}
return false;
}
// 随机生成数独题目
void generate_board(int num) {
memset(board, 0, sizeof(board));
solve_board(0, 0);
srand(time(NULL));
for (int i = 0; i < num; i++) {
int row = rand() % N;
int col = rand() % N;
while (board[row][col] == 0) {
row = rand() % N;
col = rand() % N;
}
int temp = board[row][col];
board[row][col] = 0;
vector<pair<int, int>> v;
bool has_sol = false;
for (int num = 1; num <= 9; num++) {
if (check_num(num, row, col)) {
board[row][col] = num;
if (solve_board(0, 0)) {
v.push_back(make_pair(row, col));
has_sol = true;
}
}
}
if (!has_sol) {
board[row][col] = temp;
} else {
int index = rand() % v.size();
for (int i = 0; i < v.size(); i++) {
if (i == index) continue;
board[v[i].first][v[i].second] = 0;
}
}
}
}
// 检查数独是否填满
bool is_full() {
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (board[i][j] == 0) {
return false;
}
}
}
return true;
}
// 检查数独是否填写正确
bool check_board() {
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
int num = board[i][j];
board[i][j] = 0;
if (num != 0 && !check_num(num, i, j)) {
board[i][j] = num;
return false;
}
board[i][j] = num;
}
}
return true;
}
// 用户界面
void play_game() {
while (true) {
cout << "=========================" << endl;
cout << "Welcome to Sudoku Game!" << endl;
cout << "1. New game" << endl;
cout << "2. Check answer" << endl;
cout << "3. Quit" << endl;
cout << "=========================" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
cout << "Please input the number of blanks: ";
int num;
cin >> num;
generate_board(num);
print_board();
break;
}
case 2: {
if (is_full()) {
if (check_board()) {
cout << "Congratulations! You solved the Sudoku!" << endl;
} else {
cout << "Sorry, your answer is wrong." << endl;
}
} else {
cout << "Please finish the Sudoku first." << endl;
}
break;
}
case 3: {
cout << "Goodbye!" << endl;
return;
}
default: {
cout << "Invalid input, please try again." << endl;
break;
}
}
}
}
int main() {
play_game();
return 0;
}
```
该代码实现了数独游戏的基本功能,包括生成数独题目、回溯法求解、随机生成空格、用户界面等功能。您可以按照自己的需求进行修改和完善。
阅读全文
相关推荐



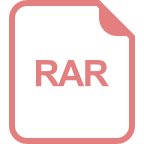

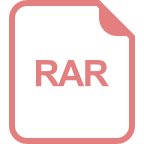


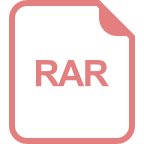

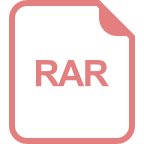




